使用 C++语言设计程序,使用二维数组初始化两个需要进行矩阵乘法运算的矩阵,同时显示可以进行 的矩阵乘法运算方式:a 矩阵的普通乘法,b 矩阵的哈达马积,c 矩阵的克 罗内克积,d 复数矩阵的普通乘法。
时间: 2023-06-19 14:06:07 浏览: 99
以下是使用C++语言设计程序,使用二维数组初始化两个需要进行矩阵乘法运算的矩阵,同时显示可以进行的矩阵乘法运算方式的代码:
```c++
#include <iostream>
using namespace std;
const int MAX = 100;
void matrixMultiplication(int matrixA[][MAX], int matrixB[][MAX], int rowA, int colA, int rowB, int colB)
{
int result[MAX][MAX];
cout << "Available Matrix Multiplication Options:" << endl;
cout << "a. Normal Multiplication" << endl;
cout << "b. Hadamard Product" << endl;
cout << "c. Kronecker Product" << endl;
cout << "d. Complex Multiplication" << endl;
char option;
cout << "Enter the option for matrix multiplication: ";
cin >> option;
switch (option)
{
case 'a':
if (colA != rowB)
{
cout << "Matrix multiplication not possible." << endl;
return;
}
for (int i = 0; i < rowA; i++)
{
for (int j = 0; j < colB; j++)
{
result[i][j] = 0;
for (int k = 0; k < colA; k++)
{
result[i][j] += matrixA[i][k] * matrixB[k][j];
}
}
}
cout << "Result of Normal Multiplication:" << endl;
for (int i = 0; i < rowA; i++)
{
for (int j = 0; j < colB; j++)
{
cout << result[i][j] << " ";
}
cout << endl;
}
break;
case 'b':
if (rowA != rowB || colA != colB)
{
cout << "Matrix multiplication not possible." << endl;
return;
}
for (int i = 0; i < rowA; i++)
{
for (int j = 0; j < colB; j++)
{
result[i][j] = matrixA[i][j] * matrixB[i][j];
}
}
cout << "Result of Hadamard Product:" << endl;
for (int i = 0; i < rowA; i++)
{
for (int j = 0; j < colB; j++)
{
cout << result[i][j] << " ";
}
cout << endl;
}
break;
case 'c':
for (int i = 0; i < rowA; i++)
{
for (int j = 0; j < colA; j++)
{
for (int k = 0; k < rowB; k++)
{
for (int l = 0; l < colB; l++)
{
result[i * rowB + k][j * colB + l] = matrixA[i][j] * matrixB[k][l];
}
}
}
}
cout << "Result of Kronecker Product:" << endl;
for (int i = 0; i < rowA * rowB; i++)
{
for (int j = 0; j < colA * colB; j++)
{
cout << result[i][j] << " ";
}
cout << endl;
}
break;
case 'd':
if (colA != rowB)
{
cout << "Matrix multiplication not possible." << endl;
return;
}
int complexA[MAX][MAX], complexB[MAX][MAX];
cout << "Enter the values of matrix A:" << endl;
for (int i = 0; i < rowA; i++)
{
for (int j = 0; j < colA; j++)
{
cout << "Enter the real and imaginary parts of element (" << i << ", " << j << "): ";
cin >> complexA[i][j] >> complexA[i][j+1];
j++;
}
}
cout << "Enter the values of matrix B:" << endl;
for (int i = 0; i < rowB; i++)
{
for (int j = 0; j < colB; j++)
{
cout << "Enter the real and imaginary parts of element (" << i << ", " << j << "): ";
cin >> complexB[i][j] >> complexB[i][j+1];
j++;
}
}
int resultReal[MAX][MAX], resultImaginary[MAX][MAX];
for (int i = 0; i < rowA; i++)
{
for (int j = 0; j < colB; j++)
{
resultReal[i][j] = 0;
resultImaginary[i][j] = 0;
for (int k = 0; k < colA; k++)
{
resultReal[i][j] += complexA[i][k] * complexB[k][j] - complexA[i][k+1] * complexB[k][j+1];
resultImaginary[i][j] += complexA[i][k] * complexB[k][j+1] + complexA[i][k+1] * complexB[k][j];
k++;
}
}
}
cout << "Result of Complex Multiplication:" << endl;
for (int i = 0; i < rowA; i++)
{
for (int j = 0; j < colB; j++)
{
cout << resultReal[i][j] << " + " << resultImaginary[i][j] << "i\t";
}
cout << endl;
}
break;
default:
cout << "Invalid option entered." << endl;
break;
}
}
int main()
{
int matrixA[MAX][MAX], matrixB[MAX][MAX];
int rowA, colA, rowB, colB;
cout << "Enter the number of rows and columns for matrix A: ";
cin >> rowA >> colA;
cout << "Enter the values of matrix A:" << endl;
for (int i = 0; i < rowA; i++)
{
for (int j = 0; j < colA; j++)
{
cin >> matrixA[i][j];
}
}
cout << "Enter the number of rows and columns for matrix B: ";
cin >> rowB >> colB;
cout << "Enter the values of matrix B:" << endl;
for (int i = 0; i < rowB; i++)
{
for (int j = 0; j < colB; j++)
{
cin >> matrixB[i][j];
}
}
matrixMultiplication(matrixA, matrixB, rowA, colA, rowB, colB);
return 0;
}
```
此代码可以接受用户输入两个矩阵的行列数和元素,并通过调用`matrixMultiplication`函数实现矩阵乘法。在该函数中,通过用户选择的选项执行不同的矩阵乘法:a矩阵的普通乘法,b矩阵的哈达马积,c矩阵的克罗内克积,d复数矩阵的普通乘法。
阅读全文
相关推荐
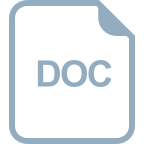




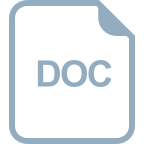
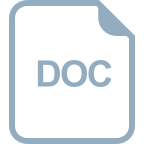
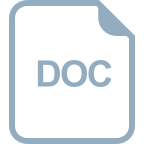
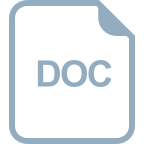

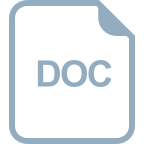
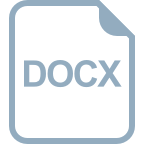
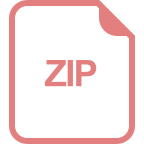
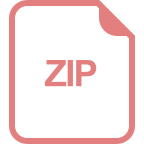