#include <iostream> #include <stack> #include <cstdlib> #include <ctime> using namespace std; const int MAXN = 100; const char WALL = '#'; const char PATH = ' '; const char START = 'S'; const char END = 'E'; const int dx[4] = { -1, 0, 1, 0 }; const int dy[4] = { 0, 1, 0, -1 }; int n, m; char maze[MAXN][MAXN]; bool vis[MAXN][MAXN]; stack<pair<int, int>> st; void init() { // 随机生成迷宫 srand(time(NULL)); n = rand() % 10 + 5; m = rand() % 10 + 5; int sx = rand() % n; int sy = rand() % m; int ex = rand() % n; int ey = rand() % m; for (int i = 0; i < n; i++) { for (int j = 0; j < m; j++) { if (i == sx && j == sy) maze[i][j] = START; else if (i == ex && j == ey) maze[i][j] = END; else if (rand() % 4 == 0) maze[i][j] = WALL; else maze[i][j] = PATH; } } } void print() { // 输出迷宫 cout << "Maze:" << endl; for (int i = 0; i < n; i++) { for (int j = 0; j < m; j++) { cout << maze[i][j] << ' '; } cout << endl; } } bool dfs(int x, int y) { // 深度优先搜索 vis[x][y] = true; st.push(make_pair(x, y)); if (maze[x][y] == END) { return true; } for (int i = 0; i < 4; i++) { int nx = x + dx[i]; int ny = y + dy[i]; if (nx >= 0 && nx < n && ny >= 0 && ny < m && maze[nx][ny] != WALL && !vis[nx][ny]) { if (dfs(nx, ny)) { return true; } } } st.pop(); return false; } void solve() { // 求解迷宫 memset(vis, false, sizeof(vis)); while (!st.empty()) st.pop(); dfs(0, 0); } void print_path() { // 输出路径 cout << "Path:" << endl; while (!st.empty()) { auto p = st.top(); st.pop(); cout << '(' << p.first << ", " << p.second << ')' << endl; } } int main() { init(); print(); solve(); print_path(); return 0; } 在这个代码的基础上,把入口S设置为迷宫左上角,出口E设置为迷宫右下角
时间: 2024-02-01 08:03:47 浏览: 27
将代码中的init函数修改如下:
```cpp
void init() {
// 随机生成迷宫
srand(time(NULL));
n = rand() % 10 + 5;
m = rand() % 10 + 5;
int sx = 0;
int sy = 0;
int ex = n - 1;
int ey = m - 1;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
if (i == sx && j == sy) maze[i][j] = START;
else if (i == ex && j == ey) maze[i][j] = END;
else if (rand() % 4 == 0) maze[i][j] = WALL;
else maze[i][j] = PATH;
}
}
}
```
这样就将入口设置为左上角,出口设置为右下角了。
相关问题
#include<iostream> #include<stack> #include<queue>
这是C++的头文件引用,分别包含了输入输出流、栈和队列的类。其中iostream头文件定义了输入输出流的类,stack头文件定义了栈的类,queue头文件定义了队列的类。以下是它们的简单使用示例:
1. iostream头文件的使用:
```c++
#include<iostream>
using namespace std;
int main() {
int num;
cin >> num; // 从控制台读取一个整数
cout << "You entered " << num << endl; // 输出读取到的整数
return 0;
}
```
2. stack头文件的使用:
```c++
#include<iostream>
#include<stack>
using namespace std;
int main() {
stack<int> s; // 定义一个int类型的栈
s.push(1); // 压入元素1
s.push(2); // 压入元素2
s.push(3); // 压入元素3
while (!s.empty()) { // 遍历栈中元素
cout << s.top() << " "; // 输出栈顶元素
s.pop(); // 弹出栈顶元素
}
return 0;
}
```
3. queue头文件的使用:
```c++
#include<iostream>
#include<queue>
using namespace std;
int main() {
queue<int> q; // 定义一个int类型的队列
q.push(1); // 入队1
q.push(2); // 入队2
q.push(3); // 入队3
while (!q.empty()) { // 遍历队列中元素
cout << q.front() << " "; // 输出队首元素
q.pop(); // 弹出队首元素
}
return 0;
}
```
include <iostream>#include <cstdlib> #include <ctime>
这是C++代码,用于包含头文件iostream、cstdlib和ctime。这些头文件提供了一些常用的函数和类,例如输入输出流、随机数生成和时间函数等。下面是一个简单的示例代码,演示了如何使用这些头文件中的函数:
```cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
// 生成随机数种子
std::srand(std::time(0));
// 生成一个随机数
int randomNumber = std::rand();
// 输出随机数
std::cout << "Random number: " << randomNumber << std::endl;
return 0;
}
```
这段代码首先使用`std::time(0)`函数获取当前时间作为随机数种子,然后使用`std::srand()`函数将种子传递给随机数生成器。接下来,使用`std::rand()`函数生成一个随机数,并使用`std::cout`输出到控制台。
相关推荐
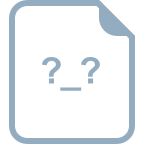













