用C语言实现题目:给定一个大小为 n 的数组,找到在数组中出现次数 大于 ⌊ n/2 ⌋ 的元素。
时间: 2024-05-10 10:21:31 浏览: 63
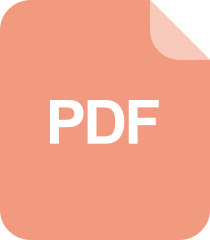
JS获取数组中出现次数最多及第二多元素的方法
一种简单的解决方法是使用哈希表。遍历整个数组,将每个元素作为键,将它们的出现次数作为值存储在哈希表中。最后再遍历一遍哈希表,找到出现次数大于 ⌊ n/2 ⌋ 的元素。
具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define HASH_SIZE 100
typedef struct node {
int key;
int count;
struct node *next;
} Node;
int hash(int key) {
return key % HASH_SIZE;
}
void insert(Node **hashTable, int key) {
int index = hash(key);
Node *p = hashTable[index];
while (p != NULL) {
if (p->key == key) {
p->count++;
return;
}
p = p->next;
}
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->key = key;
newNode->count = 1;
newNode->next = hashTable[index];
hashTable[index] = newNode;
}
int findMajority(Node **hashTable, int n) {
int threshold = n / 2;
for (int i = 0; i < HASH_SIZE; i++) {
Node *p = hashTable[i];
while (p != NULL) {
if (p->count > threshold) {
return p->key;
}
p = p->next;
}
}
return -1;
}
int main() {
int n;
printf("Enter the size of the array: ");
scanf("%d", &n);
int *a = (int *)malloc(sizeof(int) * n);
printf("Enter the elements of the array: ");
for (int i = 0; i < n; i++) {
scanf("%d", &a[i]);
}
Node **hashTable = (Node **)malloc(sizeof(Node *) * HASH_SIZE);
for (int i = 0; i < HASH_SIZE; i++) {
hashTable[i] = NULL;
}
for (int i = 0; i < n; i++) {
insert(hashTable, a[i]);
}
int majority = findMajority(hashTable, n);
if (majority != -1) {
printf("The majority element is %d\n", majority);
} else {
printf("There is no majority element\n");
}
return 0;
}
```
这里使用了一个简单的哈希函数,将键值取模,将余数作为哈希表的下标。在遍历数组时,将每个元素插入哈希表中。如果已经存在,则增加计数器;否则创建一个新的节点。在查找阶段,遍历哈希表,找到计数器大于阈值的节点即可。如果没找到,则说明没有出现次数大于 ⌊ n/2 ⌋ 的元素。
阅读全文
相关推荐
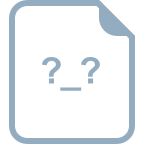
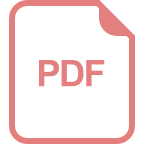
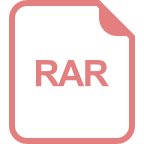
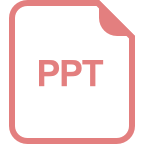
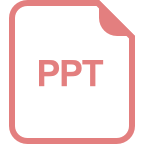
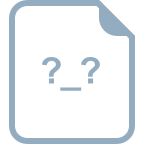
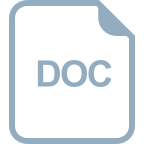
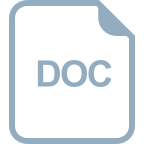
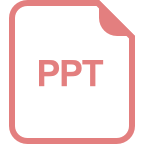
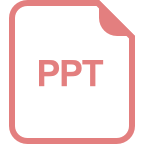
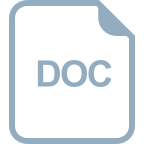
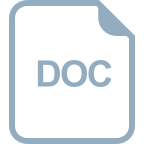