c++sort函数
时间: 2023-07-23 08:04:11 浏览: 33
C++中的`sort`函数是一个非常常用的排序函数,可以对数组或容器中的元素进行排序。其函数原型为:
```c++
template<class RandomIt>
void sort(RandomIt first, RandomIt last);
template<class RandomIt, class Compare>
void sort(RandomIt first, RandomIt last, Compare comp);
```
其中第一个函数适用于普通类型的元素,第二个函数适用于自定义类型的元素。
例如对于一个数组进行排序,可以这样写:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
int arr[] = {5, 3, 1, 4, 2};
int n = sizeof(arr) / sizeof(arr[0]);
sort(arr, arr + n);
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
```
输出结果为:`1 2 3 4 5`。
对于自定义类型,可以通过重载`operator<`来指定排序规则,例如:
```c++
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
struct Student {
string name;
int score;
bool operator<(const Student& other) const {
return score > other.score; // 按照分数从高到低排序
}
};
int main() {
vector<Student> students = {
{"Tom", 90},
{"Jerry", 80},
{"Alice", 95},
{"Bob", 85}
};
sort(students.begin(), students.end());
for (const auto& student : students) {
cout << student.name << " " << student.score << endl;
}
return 0;
}
```
输出结果为:
```
Alice 95
Tom 90
Bob 85
Jerry 80
```
阅读全文
相关推荐
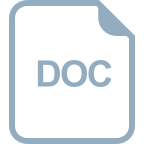
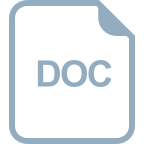
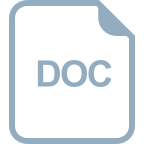
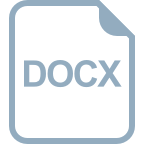
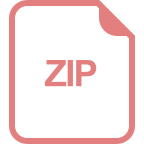
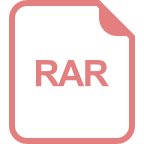
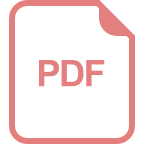
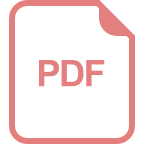
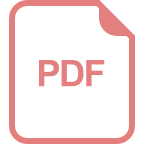
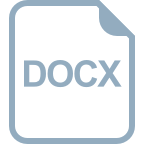
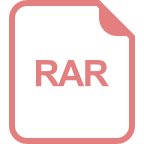
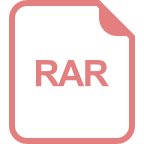
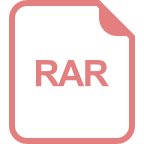
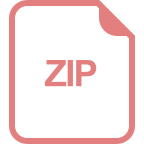
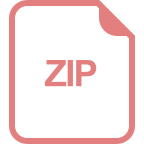
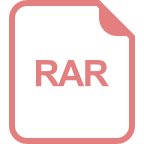
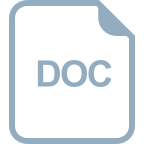
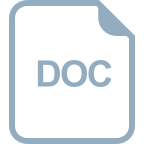