ubuntu中,a(){ echo -n "$1";return 10};a 5;echo $?; q; exit的输出结果
时间: 2024-02-25 16:53:00 浏览: 62
在 Ubuntu 中执行这段代码,输出结果如下:
```
5
10
bash: q: command not found
```
解释如下:
1. 首先定义了一个函数 `a()`,函数的作用是将函数的第一个参数输出,并且将函数的返回值设置为 10。
2. 执行命令 `a 5`,将数字 5 作为参数传递给函数 `a()`,函数输出数字 5,没有换行符,因为使用了 `-n` 选项,然后函数的返回值被设置为 10。
3. 执行命令 `echo $?`,输出上一个命令的返回值,即函数 `a()` 的返回值,所以输出的是数字 10。
4. 执行命令 `q`,由于在系统中没有定义名为 `q` 的命令,所以输出 `bash: q: command not found`。
5. 执行命令 `exit`,退出当前的 shell。
需要注意的是,在执行函数 `a()` 时,函数的返回值被设置为 10,所以在执行命令 `echo $?` 时,输出的是数字 10,而不是 0。
相关问题
Linux内ubuntu利用ncurse实现俄罗斯方块简单代码,可输出执行文件
好的,以下是一个简单的使用ncurses库实现俄罗斯方块的代码,你可以编译生成可执行文件:
```c++
#include <ncurses.h>
#include <stdlib.h>
#include <time.h>
#define ROWS 20
#define COLS 10
int block[7][4][4] = {
{{0, 0, 0, 0},
{1, 1, 1, 1},
{0, 0, 0, 0},
{0, 0, 0, 0}},
{{0, 0, 0, 0},
{0, 2, 2, 0},
{0, 2, 2, 0},
{0, 0, 0, 0}},
{{0, 0, 0, 0},
{0, 3, 3, 0},
{3, 3, 0, 0},
{0, 0, 0, 0}},
{{0, 0, 0, 0},
{4, 4, 0, 0},
{0, 4, 4, 0},
{0, 0, 0, 0}},
{{0, 0, 0, 0},
{0, 5, 5, 0},
{5, 5, 0, 0},
{0, 0, 0, 0}},
{{0, 0, 0, 0},
{6, 6, 6, 0},
{0, 6, 0, 0},
{0, 0, 0, 0}},
{{0, 0, 0, 0},
{7, 7, 7, 0},
{7, 0, 0, 0},
{0, 0, 0, 0}}
};
int board[ROWS][COLS] = {0};
int cur_block[4][4] = {0};
int cur_row = 0, cur_col = 0, cur_type = 0;
int score = 0;
void init_board()
{
int i, j;
for(i = 0; i < ROWS; i++) {
for(j = 0; j < COLS; j++) {
board[i][j] = 0;
}
}
}
void draw_block(int row, int col, int type)
{
int i, j;
for(i = 0; i < 4; i++) {
for(j = 0; j < 4; j++) {
if(block[type][i][j] != 0) {
mvprintw(row+i, col+j*2, "[]");
}
}
}
}
void erase_block(int row, int col, int type)
{
int i, j;
for(i = 0; i < 4; i++) {
for(j = 0; j < 4; j++) {
if(block[type][i][j] != 0) {
mvprintw(row+i, col+j*2, " ");
}
}
}
}
void draw_board()
{
int i, j;
for(i = 0; i < ROWS; i++) {
for(j = 0; j < COLS; j++) {
if(board[i][j] != 0) {
mvprintw(i, j*2, "[]");
}
}
}
}
void new_block()
{
cur_row = 0;
cur_col = COLS / 2 - 2;
cur_type = rand() % 7;
memcpy(cur_block, block[cur_type], sizeof(cur_block));
}
int can_move(int row, int col)
{
int i, j;
for(i = 0; i < 4; i++) {
for(j = 0; j < 4; j++) {
if(cur_block[i][j] != 0) {
int r = row + i;
int c = col + j;
if(r < 0 || r >= ROWS || c < 0 || c >= COLS || board[r][c] != 0) {
return 0;
}
}
}
}
return 1;
}
void merge_block()
{
int i, j;
for(i = 0; i < 4; i++) {
for(j = 0; j < 4; j++) {
if(cur_block[i][j] != 0) {
int r = cur_row + i;
int c = cur_col + j;
board[r][c] = cur_block[i][j];
}
}
}
}
void check_full_row()
{
int i, j;
for(i = ROWS-1; i >= 0; i--) {
int full = 1;
for(j = 0; j < COLS; j++) {
if(board[i][j] == 0) {
full = 0;
break;
}
}
if(full) {
memcpy(board[1], board[0], COLS*sizeof(int));
memset(board[0], 0, COLS*sizeof(int));
score += 10;
}
}
}
void game_over()
{
erase_block(cur_row, cur_col, cur_type);
mvprintw(ROWS/2, COLS, "Game Over! Score: %d\n", score);
refresh();
getch();
}
void move_left()
{
if(can_move(cur_row, cur_col-1)) {
erase_block(cur_row, cur_col, cur_type);
cur_col--;
draw_block(cur_row, cur_col, cur_type);
}
}
void move_right()
{
if(can_move(cur_row, cur_col+1)) {
erase_block(cur_row, cur_col, cur_type);
cur_col++;
draw_block(cur_row, cur_col, cur_type);
}
}
void move_down()
{
if(can_move(cur_row+1, cur_col)) {
erase_block(cur_row, cur_col, cur_type);
cur_row++;
draw_block(cur_row, cur_col, cur_type);
} else {
merge_block();
check_full_row();
new_block();
if(!can_move(cur_row, cur_col)) {
game_over();
exit(0);
}
}
}
void rotate()
{
int new_block[4][4] = {0};
int i, j;
for(i = 0; i < 4; i++) {
for(j = 0; j < 4; j++) {
new_block[i][j] = cur_block[3-j][i];
}
}
if(can_move(cur_row, cur_col) && can_move(cur_row, cur_col+1)) {
erase_block(cur_row, cur_col, cur_type);
memcpy(cur_block, new_block, sizeof(cur_block));
draw_block(cur_row, cur_col, cur_type);
}
}
int main()
{
srand(time(NULL));
initscr();
cbreak();
noecho();
keypad(stdscr, TRUE);
curs_set(0);
timeout(1000);
init_board();
new_block();
while(1) {
draw_board();
draw_block(cur_row, cur_col, cur_type);
char ch = getch();
switch(ch) {
case 'q':
endwin();
exit(0);
case KEY_LEFT:
move_left();
break;
case KEY_RIGHT:
move_right();
break;
case KEY_DOWN:
move_down();
break;
case ' ':
rotate();
break;
}
}
endwin();
return 0;
}
```
编译生成可执行文件的命令:
```
gcc -o tetris tetris.c -lncurses
```
运行可执行文件:
```
./tetris
```
ubuntu安装prometheus
要在Ubuntu上安装Prometheus,您可以按照以下步骤进行操作:
1.创建Prometheus服务启动配置文件:
```
cd /etc/init.d/
sudo vim prometheus
```
2.将以下内容复制到文件中:
```
#!/bin/bash
# /etc/init.d/prometheus
### BEGIN INIT INFO
# Provides: prometheus
# Required-Start: $remote_fs $syslog
# Required-Stop: $remote_fs $syslog
# Default-Start: 2 3 4 5
# Default-Stop: 0 1 6
# Short-Description: Prometheus monitoring system
# Description: Prometheus is a systems and service monitoring system.
### END INIT INFO
NAME=prometheus
PATH=/usr/local/sbin:/usr/local/bin:/sbin:/bin:/usr/sbin:/usr/bin
DAEMON=/usr/local/bin/prometheus
DAEMON_ARGS="-config.file=/etc/prometheus/prometheus.yml -storage.local.path=/var/lib/prometheus/ -web.console.templates=/etc/prometheus/consoles -web.console.libraries=/etc/prometheus/console_libraries"
PIDFILE=/var/run/$NAME.pid
USER=prometheus
GROUP=prometheus
UMASK=022
# Exit if the package is not installed
[ -x "$DAEMON" ] || exit 0
# Load the VERBOSE setting and other rcS variables
. /lib/init/vars.sh
# Define LSB log_* functions.
# Depend on lsb-base (>= 3.2-14) to ensure that this file is present
# and status_of_proc is working.
. /lib/lsb/init-functions
#
# Function that starts the daemon/service
#
do_start()
{
# Return
# 0 if daemon has been started
# 1 if daemon was already running
# 2 if daemon could not be started
start-stop-daemon --start --quiet --pidfile $PIDFILE --exec $DAEMON --test > /dev/null \
|| return 1
start-stop-daemon --start --quiet --pidfile $PIDFILE --exec $DAEMON -- \
$DAEMON_ARGS \
|| return 2
# Add code here, if necessary, that waits for the process to be ready
# to handle requests from services started subsequently which depend
# on this one. As a last resort, sleep for some time.
}
#
# Function that stops the daemon/service
#
do_stop()
{
# Return
# 0 if daemon has been stopped
# 1 if daemon was already stopped
# 2 if daemon could not be stopped
# other if a failure occurred
start-stop-daemon --stop --quiet --retry=TERM/30/KILL/5 --pidfile $PIDFILE --name $NAME
RETVAL="$?"
[ "$RETVAL" = 2 ] && return 2
# Wait for children to finish too if this is a daemon that forks
# and if the daemon is only ever run from this initscript.
# If the above conditions are not satisfied then add some other code
# that waits for the process to drop all resources that could be
# needed by services started subsequently. A last resort is to
# sleep for some time.
start-stop-daemon --stop --quiet --oknodo --retry=0/30/KILL/5 --exec $DAEMON
[ "$?" = 2 ] && return 2
# Many daemons don't delete their pidfiles when they exit.
rm -f $PIDFILE
return "$RETVAL"
}
#
# Function that sends a SIGHUP to the daemon/service
#
do_reload() {
#
# If the daemon can reload its configuration without
# restarting (for example, when it is sent a SIGHUP),
# then implement that here.
#
start-stop-daemon --stop --signal 1 --quiet --pidfile $PIDFILE --name $NAME
return 0
}
case "$1" in
start)
[ "$VERBOSE" != no ] && log_daemon_msg "Starting $NAME" "$NAME"
do_start
case "$?" in
0|1) [ "$VERBOSE" != no ] && log_end_msg 0 ;;
2) [ "$VERBOSE" != no ] && log_end_msg 1 ;;
esac
;;
stop)
[ "$VERBOSE" != no ] && log_daemon_msg "Stopping $NAME" "$NAME"
do_stop
case "$?" in
0|1) [ "$VERBOSE" != no ] && log_end_msg 0 ;;
2) [ "$VERBOSE" != no ] && log_end_msg 1 ;;
esac
;;
status)
status_of_proc "$DAEMON" "$NAME" && exit 0 || exit $?
;;
#reload|force-reload)
#
# If do_reload() is not implemented then leave this commented out
# and leave 'force-reload' as an alias for 'restart'.
#
#log_daemon_msg "Reloading $NAME" "$NAME"
#do_reload
#log_end_msg $?
#;;
restart|force-reload)
#
# If the "reload" option is implemented then remove the
# 'force-reload' alias
#
log_daemon_msg "Restarting $NAME" "$NAME"
do_stop
case "$?" in
0|1)
do_start
case "$?" in
0) log_end_msg 0 ;;
1) log_end_msg 1 ;; # Old process is still running
*) log_end_msg 1 ;; # Failed to start
esac
;;
*)
# Failed to stop
log_end_msg 1
;;
esac
;;
*)
#echo "Usage: $SCRIPTNAME {start|stop|restart|reload|force-reload}" >&2
echo "Usage: $SCRIPTNAME {start|stop|status|restart|force-reload}" >&2
exit 3
;;
esac
exit 0
```
3.保存并退出文件。
4.下载最新版本的Prometheus:
```
wget https://github.com/prometheus/prometheus/releases/download/v2.40.4/prometheus-2.40.4.linux-amd64.tar.gz
```
5.解压缩下载的文件:
```
tar -xf prometheus-2.40.4.linux-amd64.tar.gz
```
6.进入解压缩后的目录:
```
cd prometheus-2.40.4.linux-amd64
```
7.启动Prometheus服务:
```
sudo service prometheus start
```
8.检查Prometheus服务是否正在运行:
```
sudo service prometheus status
```
阅读全文
相关推荐
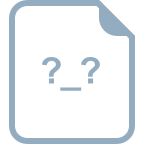
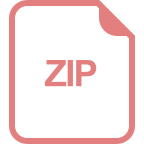
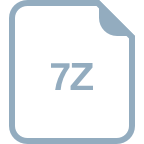
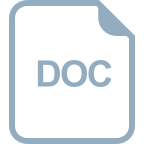
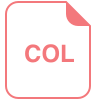
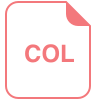
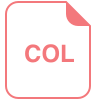
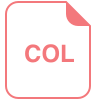






