java 结合opc ua实现订阅并且利用websocket返给前端
时间: 2023-08-05 20:41:57 浏览: 48
好的,下面是一个简单的示例代码,展示如何在Java中订阅OPC UA数据并使用WebSocket将数据返回前端:
1. 创建Java项目,添加Java WebSocket API和Eclipse Milo依赖:
```xml
<dependency>
<groupId>javax.websocket</groupId>
<artifactId>javax.websocket-api</artifactId>
<version>1.1</version>
</dependency>
<dependency>
<groupId>org.eclipse.milo</groupId>
<artifactId>milo-client-sdk</artifactId>
<version>0.6.6</version>
</dependency>
```
2. 在Java中实现WebSocket服务器,使用Java WebSocket API提供的标准库:
```java
@ServerEndpoint("/websocket")
public class WebSocketServer {
private static Set<Session> sessions = Collections.synchronizedSet(new HashSet<>());
@OnOpen
public void onOpen(Session session) {
sessions.add(session);
}
@OnClose
public void onClose(Session session) {
sessions.remove(session);
}
public static void sendMessage(String message) {
for (Session session : sessions) {
try {
session.getBasicRemote().sendText(message);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
3. 在Java中实现OPC UA订阅,使用Eclipse Milo提供的API:
```java
public class OpcUaSubscription {
private UaSubscription subscription;
private Consumer<DataValue> dataConsumer;
public OpcUaSubscription(UaClient client, NodeId nodeId, Consumer<DataValue> dataConsumer) throws Exception {
this.dataConsumer = dataConsumer;
// 创建订阅
subscription = client.getSubscriptionManager().createSubscription(1000.0).get();
// 添加数据变化监听器
UaMonitoredItem item = subscription.createMonitoredItem(
new MonitoringParameters(
nodeId,
Unsigned.uint(0),
OpcUaExtensionObject.encode(
new ExtensionObject(
new MonitoringFilter(
null,
TriggeringEvent.ALL,
Counter.none(),
ExtensionObject.encode(new DataChangeFilter(
DataChangeTrigger.StatusValue,
DeadbandType.Percent,
0.0
))
)
)
),
1000.0,
true
),
this::onDataChanged
).get();
}
private void onDataChanged(UaMonitoredItem item, MonitoredItemNotification notification) {
for (DataValue value : notification.getValue().getValue()) {
dataConsumer.accept(value);
}
}
public void close() {
subscription.delete();
}
}
```
4. 在Java中编写程序,连接OPC UA Server,订阅数据,并将数据发送至WebSocket服务器:
```java
public class Main {
public static void main(String[] args) throws Exception {
// 创建WebSocket服务器
WebSocketContainer container = ContainerProvider.getWebSocketContainer();
container.connectToServer(WebSocketServer.class, new URI("ws://localhost:8080/websocket"));
// 连接OPC UA Server
OpcUaClientConfig config = OpcUaClientConfig.builder()
.setEndpoint(new URI("opc.tcp://localhost:4840"))
.build();
try (OpcUaClient client = new OpcUaClient(config)) {
client.connect().get();
// 订阅数据
NodeId nodeId = new NodeId(2, "MyVariable");
OpcUaSubscription subscription = new OpcUaSubscription(client, nodeId, value -> {
// 发送数据至WebSocket服务器
WebSocketServer.sendMessage(value.getValue().toString());
});
// 等待程序退出
System.in.read();
subscription.close();
}
}
}
```
这段代码实现了一个简单的Java程序,连接OPC UA Server,订阅名为"MyVariable"的变量,并将变量值发送至WebSocket服务器。您需要根据实际情况修改代码,例如更改OPC UA Server的地址和端口号,更改WebSocket服务器的地址和端口号等。
相关推荐
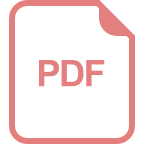
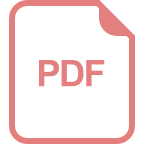
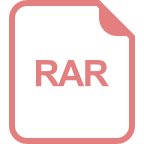














