逻辑回归的python代码
时间: 2023-10-13 21:26:12 浏览: 86
在 Python 中使用逻辑回归的代码大致如下:
```
# 导入所需的库
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
# 准备训练数据和测试数据
X = # 输入特征
y = # 标签
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 创建逻辑回归模型
model = LogisticRegression()
# 使用训练数据来训练模型
model.fit(X_train, y_train)
# 在测试数据上评估模型
accuracy = model.score(X_test, y_test)
# 输出结果
print("Accuracy: ", accuracy)
```
注意,这仅仅是一个示例。在实际使用中,您可能还需要进行调整,例如调整模型的参数或者使用其他的数据预处理技术。
相关问题
多分类逻辑回归python代码
多分类逻辑回归的Python代码可以使用scikit-learn库中的LogisticRegression类来实现。在该类中,我们可以通过设置参数solver为'multinomial'来指定使用multinomial模式进行多分类。以下是一个简单的示例代码:
```
from sklearn.linear_model import LogisticRegression
import numpy as np
# 准备数据
X = # 输入特征
y = # 输出标签
# 创建逻辑回归模型
model = LogisticRegression(solver='multinomial')
# 模型训练
model.fit(X, y)
# 预测新样本
new_sample = # 新样本数据
prediction = model.predict(new_sample)
# 打印预测结果
print(prediction)
```
在这个例子中,我们使用LogisticRegression类创建了一个多分类逻辑回归模型,并使用fit方法对模型进行训练。然后,我们可以使用predict方法对新样本进行预测,并打印预测结果。
逻辑回归模型python代码
逻辑回归是一种常用的分类算法,在Python中可以使用原生Python来实现逻辑回归模型。以下是一个简单的逻辑回归模型的Python代码示例:
```python
import numpy as np
def sigmoid(z):
return 1 / (1 + np.exp(-z))
def logistic_regression(X, y, num_iterations, learning_rate):
m, n = X.shape
theta = np.zeros((n, 1))
for i in range(num_iterations):
z = np.dot(X, theta)
h = sigmoid(z)
gradient = np.dot(X.T, (h - y)) / m
theta -= learning_rate * gradient
return theta
# 使用示例
X = np.array([[1, 2], [3, 4], [5, 6]])
y = np.array([[0], [1], [0]])
num_iterations = 1000
learning_rate = 0.01
theta = logistic_regression(X, y, num_iterations, learning_rate)
print("逻辑回归模型的参数(theta):")
print(theta)
```
阅读全文
相关推荐
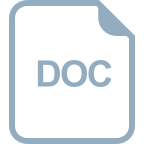
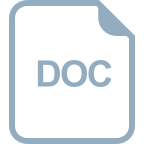
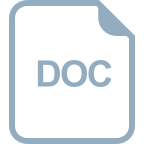
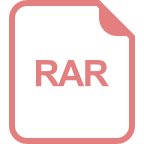
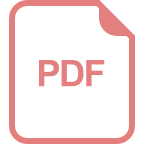
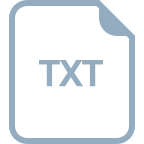
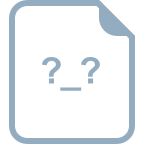
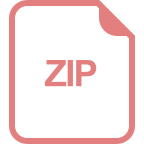
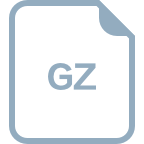
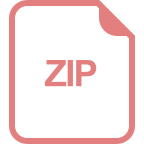
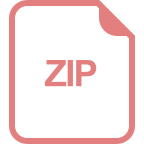
