C++求解迷宫,用链栈
时间: 2024-06-19 07:04:29 浏览: 9
以下是使用链栈求解迷宫的C++代码,其中使用了深度优先搜索算法(DFS):
```c++
#include <iostream>
#include <stack>
using namespace std;
const int MAXSIZE = 50; // 假设迷宫最大为50*50的长方阵
int n, m; // 迷宫的行数和列数
int maze[MAXSIZE][MAXSIZE]; // 存储迷宫信息,0表示可以通过,1表示障碍物
bool vis[MAXSIZE][MAXSIZE]; // 标记每个位置是否到达过
struct Pos { // 定义位置结构体,包含行和列
int x, y;
Pos(int xx = 0, int yy = 0) : x(xx), y(yy) {}
};
stack<Pos> s; // 定义存储位置的栈
// 判断当前位置是否可达
bool checkPos(const Pos& p) {
if (p.x < 0 || p.x >= n || p.y < 0 || p.y >= m) return false; // 越界
if (maze[p.x][p.y] == 1 || vis[p.x][p.y]) return false; // 障碍或已到达过
return true;
}
// 深度优先搜索
bool dfs() {
Pos start(0, 0), end(n - 1, m - 1); // 起点和终点
s.push(start); // 将起点入栈
vis[start.x][start.y] = true; // 标记起点为已到达过
while (!s.empty()) { // 当栈不为空时
Pos cur = s.top(); s.pop(); // 取出栈顶元素
if (cur.x == end.x && cur.y == end.y) return true; // 到达终点,搜索成功
for (int i = 0; i < 4; ++i) { // 四个方向依次搜索
Pos next(cur.x + dx[i], cur.y + dy[i]); // 计算下一个位置
if (checkPos(next)) { // 如果下一个位置可达
s.push(next); // 将其入栈
vis[next.x][next.y] = true; // 标记已到达
}
}
}
return false; // 栈为空,搜索失败
}
int main() {
cin >> n >> m;
for (int i = 0; i < n; ++i) {
for (int j = 0; j < m; ++j) {
cin >> maze[i][j];
vis[i][j] = false;
}
}
if (dfs()) cout << "Yes\n";
else cout << "No\n";
return 0;
}
```
相关推荐
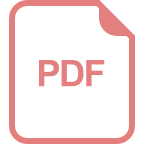














