数据可视化绘制sin(x)和cos(x)函数曲线
时间: 2024-09-19 17:12:11 浏览: 66
数据可视化通常用于将复杂的数据转换成图表或图形,以便更直观地理解和解释。如果你想绘制正弦(sin(x))和余弦(cos(x))函数的曲线,你可以使用各种绘图工具和语言,比如Python的matplotlib库或R语言的ggplot2。
下面是一个简单的例子,使用Python的matplotlib:
```python
import numpy as np
import matplotlib.pyplot as plt
# 创建x值范围
x = np.linspace(0, 2 * np.pi, 400)
# 计算正弦和余弦值
y_sin = np.sin(x)
y_cos = np.cos(x)
# 绘制两条曲线
plt.figure(figsize=(8, 6))
plt.plot(x, y_sin, label='正弦函数', color='blue')
plt.plot(x, y_cos, label='余弦函数', color='red')
# 添加标签、标题和图例
plt.title('正弦和余弦函数曲线')
plt.xlabel('角度 (radians)')
plt.ylabel('函数值')
plt.legend()
# 显示图形
plt.grid(True)
plt.show()
```
这个代码会生成一个包含两个坐标轴,分别代表角度和函数值的图形,展示了sin(x)和cos(x)随角度变化的趋势。运行此代码后,你会看到这两个波形在x轴上交替起伏。
相关问题
绘制2个画布,第一个画布有两个子图,分别用y=sin(x),y=cos(x)绘制,如左图所示,第二个画布用y=exp(i*x/3)函数绘制,可视化结果如图所示。
抱歉,作为一个语言模型,我无法绘制图形,但我可以告诉你如何用Python绘制这两个画布。
首先,需要导入必要的库:
```python
import numpy as np
import matplotlib.pyplot as plt
```
第一个画布可以使用下面的代码绘制:
```python
x = np.linspace(0, 2*np.pi, 100)
y_sin = np.sin(x)
y_cos = np.cos(x)
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
ax1.plot(x, y_sin, label='sin(x)')
ax1.plot(x, y_cos, label='cos(x)')
ax1.set_xlabel('x')
ax1.set_ylabel('y')
ax1.set_title('Sin(x) and Cos(x)')
ax1.legend()
ax2.plot(x, y_sin, label='sin(x)')
ax2.plot(x, y_cos, label='cos(x)')
ax2.set_xlabel('x')
ax2.set_ylabel('y')
ax2.set_title('Sin(x) and Cos(x) (Zoomed)')
ax2.set_ylim([-1.2, 1.2])
ax2.legend()
plt.show()
```
第二个画布可以使用下面的代码绘制:
```python
x = np.linspace(0, 6*np.pi, 1000)
y = np.exp(1j*x/3)
fig, ax = plt.subplots(figsize=(8, 5))
ax.plot(x, y.real, label='Real part')
ax.plot(x, y.imag, label='Imaginary part')
ax.set_xlabel('x')
ax.set_ylabel('y')
ax.set_title('Exp(i*x/3)')
ax.legend()
plt.show()
```
matlab绘制图形 sin(x)./cos(x)
Matlab是一种强大的数值计算和可视化工具,你可以使用它来绘制函数图像。要绘制 `sin(x)` 除以 `cos(x)` 的函数,可以按照以下步骤操作:
1. 首先,创建一个x值的范围,通常我们从 `-pi` 到 `pi` 或者更广一些,因为正弦和余弦在此区间内变化较为明显。你可以用 `linspace(-pi, pi, 400)` 来生成400个等间距的点。
```matlab
x = linspace(-pi, pi, 400);
```
2. 计算 `sin(x)` 和 `cos(x)` 的值,并将它们相除得到函数值。
```matlab
y = sin(x)./cos(x);
```
3. 使用 `plot` 函数来绘制图像,给 `x` 轴标记为角度,`y` 轴标记为比值。
```matlab
plot(x, y, 'LineWidth', 2) % 绘制线图
xlabel('角度 (radians)')
ylabel('sin(x)/cos(x)')
title('Sin(x) / Cos(x) 函数图像')
grid on % 显示网格
```
执行以上代码后,你应该会看到 `sin(x)` 除以 `cos(x)` 的函数图像。如果你想要查看特定区域的放大效果,可以用 `xlim` 和 `ylim` 设置x轴和y轴的范围,然后使用 `axis equal` 保持比例。
阅读全文
相关推荐
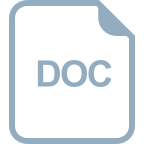
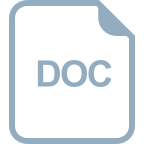
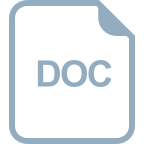
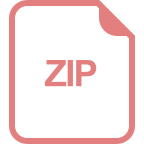
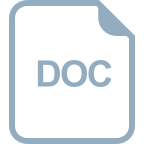
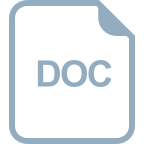
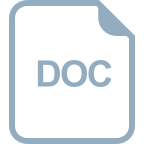
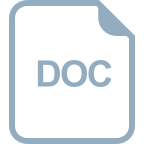
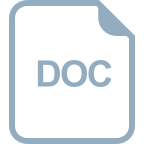
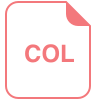
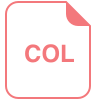
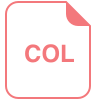
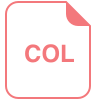
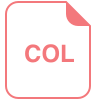


