使用 java实现顺序表的基本操作
时间: 2024-06-14 21:07:44 浏览: 92
以下是使用Java实现顺序表的基本操作的示例代码:
1. 初始化顺序表
```java
public class SeqList {
private int[] elem; // 存储数据的数组
private int usedSize; // 顺序表中已使用的元素个数
public SeqList(int capacity) {
this.elem = new int[capacity];
this.usedSize = 0;
}
}
```
2. 在顺序表末尾插入元素
```java
public void add(int data) {
if (this.usedSize == this.elem.length) {
// 扩容
this.elem = Arrays.copyOf(this.elem, this.elem.length * 2);
}
this.elem[this.usedSize++] = data;
}
```
3. 删除指定位置的元素
```java
public void remove(int index) {
if (index < 0 || index >= this.usedSize) {
throw new IndexOutOfBoundsException("Index out of range");
}
for (int i = index; i < this.usedSize - 1; i++) {
this.elem[i] = this.elem[i + 1];
}
this.usedSize--;
}
```
4. 获取指定位置的元素
```java
public int get(int index) {
if (index < 0 || index >= this.usedSize) {
throw new IndexOutOfBoundsException("Index out of range");
}
return this.elem[index];
}
```
5. 修改指定位置的元素
```java
public void set(int index, int data) {
if (index < 0 || index >= this.usedSize) {
throw new IndexOutOfBoundsException("Index out of range");
}
this.elem[index] = data;
}
```
6. 获取顺序表的长度
```java
public int size() {
return this.usedSize;
}
```
7. 打印顺序表的元素
```java
public void display() {
for (int i = 0; i < this.usedSize; i++) {
System.out.print(this.elem[i] + " ");
}
System.out.println();
}
```
阅读全文
相关推荐
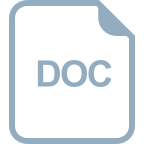
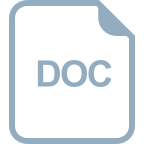
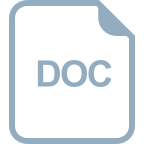
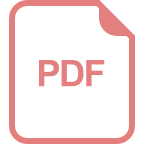
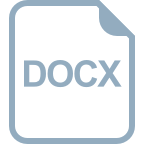
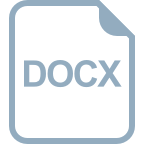
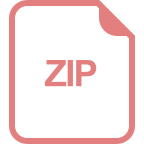
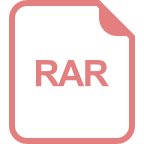
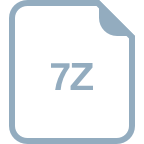
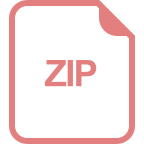
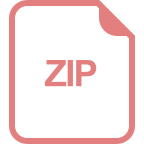
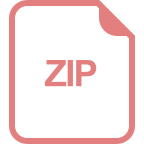
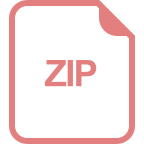
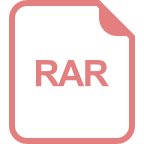
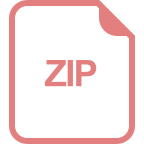
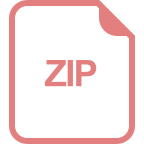
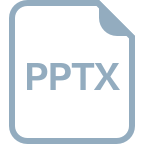
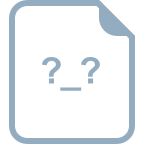
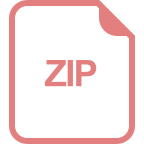