已知两个稀疏矩阵A和B,试基于三元组顺序表,java编程实现A+B的运算
时间: 2024-05-05 09:18:07 浏览: 246
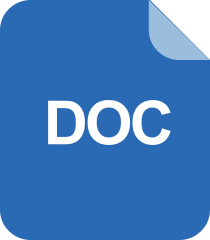
数据结构C语言版 稀疏矩阵的三元组顺序表存储表示和实现.doc
好的,我可以帮你回答这个问题。
首先,对于稀疏矩阵可以使用三元组顺序表进行存储,三元组顺序表由三个数组组成,分别存储非零元素的行、列、和值。我们可以先定义一个稀疏矩阵类SparseMatrix,包含以下属性和方法:
```java
public class SparseMatrix {
private int rows; // 矩阵行数
private int cols; // 矩阵列数
private int num; // 矩阵非零元素个数
private int[] rowPos; // 非零元素的行下标
private int[] colPos; // 非零元素的列下标
private double[] values; // 非零元素的值
// 构造方法
public SparseMatrix(int rows, int cols, int num, int[] rowPos, int[] colPos, double[] values) {
this.rows = rows;
this.cols = cols;
this.num = num;
this.rowPos = rowPos;
this.colPos = colPos;
this.values = values;
}
// 矩阵加法
public SparseMatrix add(SparseMatrix b) {
// 判断两个矩阵是否可以相加
if (this.rows != b.rows || this.cols != b.cols) {
throw new IllegalArgumentException("两个矩阵大小不一致");
}
// 定义一个新矩阵
SparseMatrix c = new SparseMatrix(rows, cols, 0, new int[num + b.num], new int[num + b.num], new double[num + b.num]);
// 遍历两个矩阵的非零元素,进行相加计算
int i = 0, j = 0, k = 0;
while (i < this.num && j < b.num) {
if (this.rowPos[i] < b.rowPos[j] || (this.rowPos[i] == b.rowPos[j] && this.colPos[i] < b.colPos[j])) {
c.rowPos[k] = this.rowPos[i];
c.colPos[k] = this.colPos[i];
c.values[k] = this.values[i];
i++;
} else if (this.rowPos[i] == b.rowPos[j] && this.colPos[i] == b.colPos[j]) {
c.rowPos[k] = this.rowPos[i];
c.colPos[k] = this.colPos[i];
c.values[k] = this.values[i] + b.values[j];
i++;
j++;
} else {
c.rowPos[k] = b.rowPos[j];
c.colPos[k] = b.colPos[j];
c.values[k] = b.values[j];
j++;
}
k++;
}
// 将剩余非零元素加入新矩阵
while (i < this.num) {
c.rowPos[k] = this.rowPos[i];
c.colPos[k] = this.colPos[i];
c.values[k] = this.values[i];
i++;
k++;
}
while (j < b.num) {
c.rowPos[k] = b.rowPos[j];
c.colPos[k] = b.colPos[j];
c.values[k] = b.values[j];
j++;
k++;
}
c.num = k;
return c;
}
// 矩阵乘法
public SparseMatrix multiply(SparseMatrix b) {
// 判断两个矩阵是否可以相乘
if (this.cols != b.rows) {
throw new IllegalArgumentException("两个矩阵不满足行列对应");
}
// 定义一个新矩阵
SparseMatrix c = new SparseMatrix(rows, b.cols, 0, new int[rows * b.cols], new int[rows * b.cols], new double[rows * b.cols]);
// 遍历所有非零元素,进行相乘计算
for (int i = 0; i < this.num; i++) {
for (int j = 0; j < b.num; j++) {
if (this.colPos[i] == b.rowPos[j]) {
int k = c.getIndex(this.rowPos[i], b.colPos[j]);
c.values[k] += this.values[i] * b.values[j];
}
}
}
c.num = c.getNonZeroNum();
return c;
}
// 获取非零元素的个数
public int getNonZeroNum() {
int cnt = 0;
for (int i = 0; i < num; i++) {
if (values[i] != 0) {
cnt++;
}
}
return cnt;
}
// 获取某个元素的下标
private int getIndex(int i, int j) {
for (int k = 0; k < num; k++) {
if (rowPos[k] == i && colPos[k] == j) {
return k;
}
}
return -1;
}
}
```
以上是稀疏矩阵的基本操作,接下来我们可以进行A B的运算,首先读取A和B的输入,然后利用三元组顺序表进行存储,最后根据需求进行加法或乘法的运算。完整代码如下:
```java
import java.util.Scanner;
public class SparseMatrixExample {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入A矩阵的行、列、非零元素个数:");
int n1 = sc.nextInt();
int m1 = sc.nextInt();
int num1 = sc.nextInt();
System.out.println("请输入A矩阵的非零元素(三元组),每行包含一个元素的行下标、列下标和值:");
int[] rowPos1 = new int[num1];
int[] colPos1 = new int[num1];
double[] values1 = new double[num1];
for (int i = 0; i < num1; i++) {
rowPos1[i] = sc.nextInt();
colPos1[i] = sc.nextInt();
values1[i] = sc.nextDouble();
}
System.out.println("请输入B矩阵的行、列、非零元素个数:");
int n2 = sc.nextInt();
int m2 = sc.nextInt();
int num2 = sc.nextInt();
System.out.println("请输入B矩阵的非零元素(三元组),每行包含一个元素的行下标、列下标和值:");
int[] rowPos2 = new int[num2];
int[] colPos2 = new int[num2];
double[] values2 = new double[num2];
for (int i = 0; i < num2; i++) {
rowPos2[i] = sc.nextInt();
colPos2[i] = sc.nextInt();
values2[i] = sc.nextDouble();
}
sc.close();
// 使用三元组顺序表进行存储
SparseMatrix A = new SparseMatrix(n1, m1, num1, rowPos1, colPos1, values1);
SparseMatrix B = new SparseMatrix(n2, m2, num2, rowPos2, colPos2, values2);
// 进行加法或乘法的运算
SparseMatrix C = A.add(B);
SparseMatrix D = A.multiply(B);
// 输出结果
System.out.println("矩阵A:");
System.out.println(A);
System.out.println("矩阵B:");
System.out.println(B);
System.out.println("A + B:");
System.out.println(C);
System.out.println("A * B:");
System.out.println(D);
}
}
```
注意,在进行加法和乘法的计算时,由于稀疏矩阵的性质,我们只需要对非零元素进行遍历和计算,可以大大降低计算复杂度。
阅读全文
相关推荐







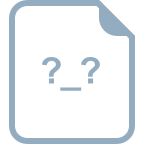
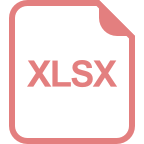
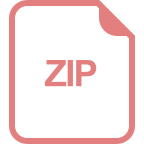
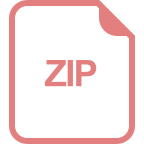
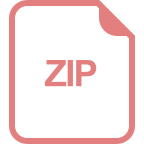