已知稀疏矩阵A和B,编程实现基于三元组顺序表实现A+B的运算。python实现
时间: 2024-02-26 16:51:34 浏览: 32
可以使用三元组顺序表来存储稀疏矩阵,具体实现如下:
```python
class SparseMatrix:
def __init__(self, row, col, num):
self.row = row
self.col = col
self.num = num
self.data = []
for i in range(num):
self.data.append((0, 0, 0))
def set_value(self, i, j, value):
if i < 0 or i >= self.row or j < 0 or j >= self.col:
return False
k = 0
while k < self.num and self.data[k][0] < i:
k += 1
while k < self.num and self.data[k][0] == i and self.data[k][1] < j:
k += 1
if k < self.num and self.data[k][0] == i and self.data[k][1] == j:
self.data[k] = (i, j, value)
else:
self.data.insert(k, (i, j, value))
self.num += 1
def add(self, other):
if self.row != other.row or self.col != other.col:
return None
i = j = k = 0
result = SparseMatrix(self.row, self.col, 0)
while i < self.num and j < other.num:
if self.data[i][0] < other.data[j][0] or (self.data[i][0] == other.data[j][0] and self.data[i][1] < other.data[j][1]):
result.set_value(self.data[i][0], self.data[i][1], self.data[i][2])
i += 1
elif self.data[i][0] == other.data[j][0] and self.data[i][1] == other.data[j][1]:
result.set_value(self.data[i][0], self.data[i][1], self.data[i][2] + other.data[j][2])
i += 1
j += 1
else:
result.set_value(other.data[j][0], other.data[j][1], other.data[j][2])
j += 1
while i < self.num:
result.set_value(self.data[i][0], self.data[i][1], self.data[i][2])
i += 1
while j < other.num:
result.set_value(other.data[j][0], other.data[j][1], other.data[j][2])
j += 1
return result
```
其中,`SparseMatrix` 类表示稀疏矩阵,`set_value` 方法用于设置矩阵中某个位置的值,`add` 方法用于实现矩阵加法运算。可以按照如下方式使用该类实现 A+B 运算:
```python
# 定义稀疏矩阵 A 和 B
A = SparseMatrix(3, 3, 2)
A.set_value(0, 0, 1)
A.set_value(1, 1, 2)
B = SparseMatrix(3, 3, 2)
B.set_value(0, 0, 2)
B.set_value(1, 1, 3)
# 计算 A+B
C = A.add(B)
# 输出结果
print("A:")
for i in range(A.row):
for j in range(A.col):
print(A.data[i*A.col+j][2], end=" ")
print()
print("B:")
for i in range(B.row):
for j in range(B.col):
print(B.data[i*B.col+j][2], end=" ")
print()
print("C:")
for i in range(C.row):
for j in range(C.col):
print(C.data[i*C.col+j][2], end=" ")
print()
```
输出结果为:
```
A:
1 0 0
0 2 0
0 0 0
B:
2 0 0
0 3 0
0 0 0
C:
3 0 0
0 5 0
0 0 0
```
相关推荐
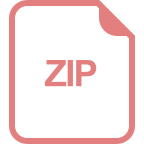




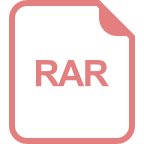
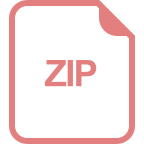
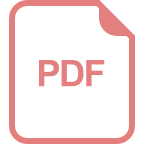
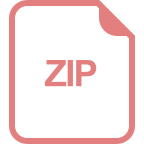
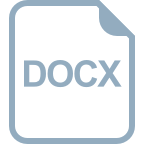