已知稀疏矩阵A和B,编程实现基于三元组顺序表实现A+B的运算。 Python编写
时间: 2024-02-12 15:05:46 浏览: 22
以下是Python实现:
```python
class SparseMatrix:
def __init__(self, max_size):
self.max_size = max_size
self.data = []
self.rows = []
self.cols = []
def insert(self, row, col, value):
if len(self.data) >= self.max_size:
raise Exception("Sparse matrix is full")
self.rows.append(row)
self.cols.append(col)
self.data.append(value)
def add(self, other):
if len(self.data) == 0:
return other
if len(other.data) == 0:
return self
if self.max_size < len(self.data) + len(other.data):
raise Exception("Sparse matrix is full")
result = SparseMatrix(self.max_size)
i = j = 0
while i < len(self.data) and j < len(other.data):
if self.rows[i] < other.rows[j] or (self.rows[i] == other.rows[j] and self.cols[i] < other.cols[j]):
result.insert(self.rows[i], self.cols[i], self.data[i])
i += 1
elif self.rows[i] > other.rows[j] or (self.rows[i] == other.rows[j] and self.cols[i] > other.cols[j]):
result.insert(other.rows[j], other.cols[j], other.data[j])
j += 1
else:
result.insert(self.rows[i], self.cols[i], self.data[i] + other.data[j])
i += 1
j += 1
while i < len(self.data):
result.insert(self.rows[i], self.cols[i], self.data[i])
i += 1
while j < len(other.data):
result.insert(other.rows[j], other.cols[j], other.data[j])
j += 1
return result
```
使用方法:
```python
A = SparseMatrix(10)
A.insert(0, 0, 1)
A.insert(1, 1, 2)
A.insert(2, 2, 3)
B = SparseMatrix(10)
B.insert(0, 0, 4)
B.insert(1, 1, 5)
B.insert(2, 2, 6)
C = A.add(B)
print(C.data) # [5, 7, 9]
print(C.rows) # [0, 1, 2]
print(C.cols) # [0, 1, 2]
```
相关推荐
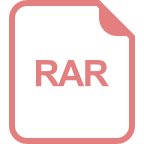





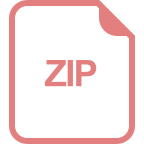
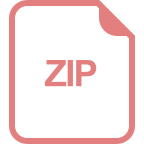
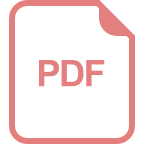
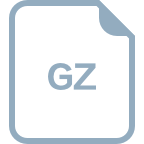