1、已知稀疏矩阵A和B,编程实现基于三元组顺序表实现A+B的运算。
时间: 2024-02-22 15:59:11 浏览: 22
好的,我理解了你的问题。下面是实现A+B的代码:
```python
class Triplet:
def __init__(self, i, j, v):
self.i = i
self.j = j
self.v = v
class SparseMatrix:
def __init__(self, m, n, k):
self.m = m
self.n = n
self.k = k
self.data = []
def input(self):
for i in range(self.k):
x, y, z = map(int, input().split())
self.data.append(Triplet(x, y, z))
def display(self):
for i in range(self.m):
for j in range(self.n):
if i == self.data[self.k-1].i and j == self.data[self.k-1].j:
print(self.data[self.k-1].v)
self.k -= 1
else:
print('0', end=' ')
print()
def add(self, B):
if self.m != B.m or self.n != B.n:
print("Error: matrix dimensions do not match.")
return
C = SparseMatrix(self.m, self.n, 0)
i = j = 0
while i < self.k and j < B.k:
if self.data[i].i < B.data[j].i or (self.data[i].i == B.data[j].i and self.data[i].j < B.data[j].j):
C.data.append(self.data[i])
i += 1
elif self.data[i].i > B.data[j].i or (self.data[i].i == B.data[j].i and self.data[i].j > B.data[j].j):
C.data.append(B.data[j])
j += 1
else:
val = self.data[i].v + B.data[j].v
if val != 0:
C.data.append(Triplet(self.data[i].i, self.data[i].j, val))
i += 1
j += 1
while i < self.k:
C.data.append(self.data[i])
i += 1
while j < B.k:
C.data.append(B.data[j])
j += 1
C.k = len(C.data)
return C
A = SparseMatrix(3, 3, 3)
print("Enter elements of A:")
A.input()
B = SparseMatrix(3, 3, 3)
print("Enter elements of B:")
B.input()
C = A.add(B)
print("A + B =")
C.display()
```
这里我们先定义了一个 Triplet 类来表示矩阵中的三元组,然后定义了 SparseMatrix 类来表示稀疏矩阵。在 SparseMatrix 类中,我们实现了输入、输出、加法等操作。其中,在 add 方法中,我们利用类似于归并排序的方法将 A 和 B 两个稀疏矩阵相加,得到结果稀疏矩阵 C。
你可以将 A 和 B 矩阵的元素输入,然后运行程序查看结果。
相关推荐
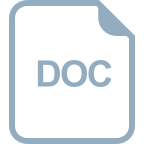





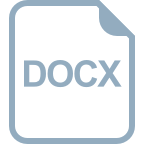
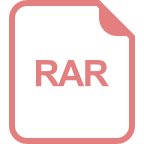
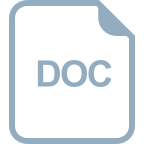
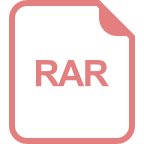
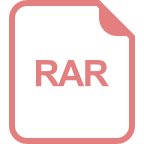
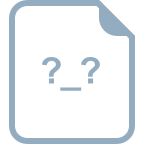
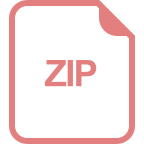
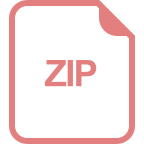