C输出工资最高的员工姓名结构体
时间: 2024-08-12 14:09:01 浏览: 41
在C语言中,我们可以使用结构体(struct)来表示员工的信息,包括姓名和工资。假设我们有一个名为`Employee`的结构体,其中包含`name`(姓名)和`salary`(工资)两个成员。为了找出工资最高的员工姓名,我们需要在结构体中定义一个比较函数或者提供一个成员函数来获取最高工资的员工。
下面是一个简单的结构体定义和示例:
```c
#include <stdio.h>
#include <string.h>
// 定义结构体
typedef struct {
char name[50]; // 员工姓名
double salary; // 员工工资
} Employee;
// 比较函数,用于找出最高工资的员工
Employee* find_highest_salary(Employee employees[], int num_employees) {
if (num_employees == 0) {
printf("No employees to compare.\n");
return NULL;
}
Employee* max_employee = &employees;
for (int i = 1; i < num_employees; i++) {
if (employees[i].salary > max_employee->salary) {
max_employee = &employees[i];
}
}
return max_employee;
}
// 输出工资最高的员工姓名
void print_highest_salary_employee(Employee* highest, int num_employees) {
if (highest != NULL) {
printf("The employee with the highest salary is: %s\n", highest->name);
} else {
printf("No employee has a salary.\n");
}
}
int main() {
// 创建一个员工数组并初始化
Employee employees[] = {
{"Alice", 50000},
{"Bob", 75000},
{"Charlie", 60000},
{"David", 80000},
};
int num_employees = sizeof(employees) / sizeof(employees);
// 找出并打印最高工资的员工
Employee* highest_salary_employee = find_highest_salary(employees, num_employees);
print_highest_salary_employee(highest_salary_employee, num_employees);
return 0;
}
```
阅读全文
相关推荐
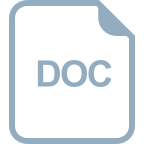
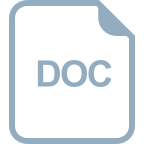
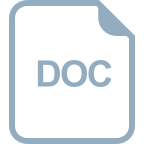















