数据结构折半查找二叉排序树
时间: 2023-12-27 07:25:43 浏览: 45
折半查找二叉排序树,也称为二叉搜索树,是一种常用的数据结构,用于快速查找和插入元素。它具有以下特点:
1. 左子树上的所有节点的值都小于根节点的值。
2. 右子树上的所有节点的值都大于根节点的值。
3. 左右子树也分别为二叉搜索树。
构建折半查找二叉排序树的方法如下:
1. 将第一个元素作为根节点。
2. 从第二个元素开始,依次与根节点比较大小。
3. 如果小于根节点,则将其作为左子树的根节点,并继续与左子树的节点比较。
4. 如果大于根节点,则将其作为右子树的根节点,并继续与右子树的节点比较。
5. 重复步骤3和4,直到所有元素都插入到树中。
以下是一个示例代码,演示了如何构建一个折半查找二叉排序树:
```python
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def insert(root, value):
if root is None:
return Node(value)
if value < root.value:
root.left = insert(root.left, value)
else:
root.right = insert(root.right, value)
return root
def inorder(root):
if root:
inorder(root.left)
print(root.value, end=" ")
inorder(root.right)
# 构建折半查找二叉排序树
arr = [5, 3, 7, 2, 4, 6, 8]
root = None
for i in arr:
root = insert(root, i)
# 中序遍历折半查找二叉排序树
inorder(root)
```
输出结果为:2 3 4 5 6 7 8
相关推荐
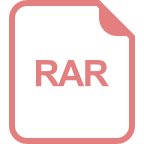
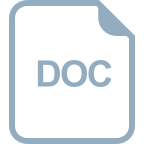
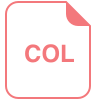














