写出一个可以进行四则运算,包括括号,小数点的c++程序
时间: 2024-02-28 09:55:23 浏览: 55
以下是一个可以进行四则运算,包括括号,小数点的c++程序:
```c++
#include <iostream>
#include <stack>
#include <string>
#include <cctype>
#include <cmath>
using namespace std;
bool is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
int priority(char c) {
if (c == '+' || c == '-') {
return 1;
} else if (c == '*' || c == '/') {
return 2;
} else {
return 0;
}
}
double calculate(double a, double b, char op) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
return a / b;
default:
return 0;
}
}
double evaluate(string expression) {
stack<double> nums;
stack<char> ops;
for (int i = 0; i < expression.length(); i++) {
char c = expression[i];
if (isdigit(c) || c == '.') {
string num_str;
num_str += c;
while (i < expression.length() - 1 && (isdigit(expression[i + 1]) || expression[i + 1] == '.')) {
num_str += expression[i + 1];
i++;
}
double num = stod(num_str);
nums.push(num);
} else if (c == '(') {
ops.push(c);
} else if (c == ')') {
while (ops.top() != '(') {
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
double result = calculate(a, b, op);
nums.push(result);
}
ops.pop();
} else if (is_operator(c)) {
while (!ops.empty() && priority(ops.top()) >= priority(c)) {
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
double result = calculate(a, b, op);
nums.push(result);
}
ops.push(c);
}
}
while (!ops.empty()) {
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
double result = calculate(a, b, op);
nums.push(result);
}
return nums.top();
}
int main() {
string expression;
cout << "请输入一个表达式:" << endl;
cin >> expression;
double result = evaluate(expression);
cout << "结果为:" << result << endl;
return 0;
}
```
可以通过输入一个表达式来计算结果,例如:
```
请输入一个表达式:
(1+2)*3-4/2
结果为:7
```
注意:在输入表达式时,请确保每个数字和操作符之间用空格隔开,否则程序可能会出现错误。
阅读全文
相关推荐
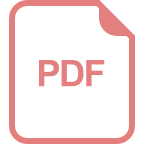
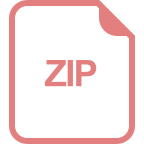
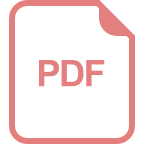
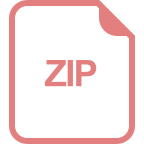
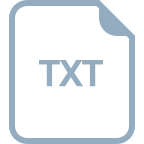
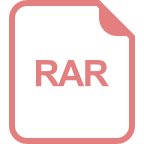
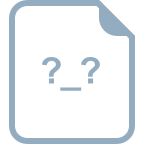
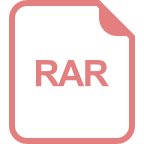
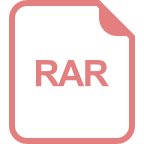
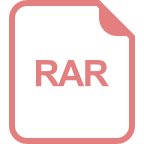
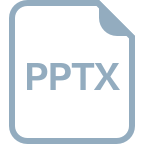
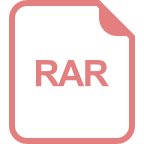
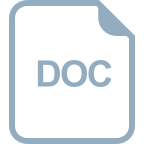
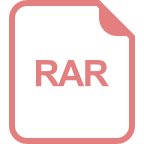
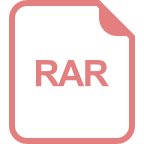
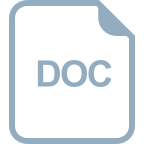