编写一个 shell 程序实现以下功能: [root@localhost ~]# Please,input a number: [root@localhost ~]# 5 (回车) 5 4 3 2 1 4 3 2 1 3 2 1 2 1 1 [root@localhost ~]# Please,input a number: [root@localhost ~]#0 (回车) [root@localhost ~]# 说明:1)程序会一直运行,直到输入0为止。 2)如果输入的是非数字,提示 Usage Error。
时间: 2024-02-16 07:03:32 浏览: 81
好的,以下是实现该功能的 shell 程序代码:
```shell
#!/bin/bash
while true
do
read -p "Please,input a number: " num
if ! [[ "$num" =~ ^[0-9]+$ ]] && [ "$num" != "0" ]; then
echo "Usage Error."
elif [ "$num" = "0" ]; then
break
else
for (( i=$num; i>=1; i-- ))
do
for (( j=$i; j>=1; j-- ))
do
echo -n "$j "
done
done
echo ""
fi
done
```
解释一下代码:
1. 使用 `while true` 实现程序一直运行。
2. 使用 `read` 获取用户输入的数字。
3. 判断输入是否为非数字或者0,如果是,则输出错误提示。
4. 如果输入为0,则退出程序。
5. 如果输入为数字,则使用两个 `for` 循环嵌套,从输入数字开始倒序输出数字,每行输出一个数字,每次输出后换行。
相关问题
、编写一个shell程序实现以下功能:[root@localhost ~]# ./run.shInput a list of number:12345(回车)The result is 120Input a list of number:q(回车)[root@localhost ~]#说明: 1) 程序会一直运行,直到输入q为止。 2需要定义一个函数来实现累乘功能。 假设用户输入的内容都是数值。
可以使用如下的脚本实现:
```bash
#!/bin/bash
function multiply() {
local res=1
for n in $@; do
res=$((res * n))
done
echo $res
}
while true; do
read -p "Input a list of number: " input
if [ "$input" = "q" ]; then
break
fi
result=$(multiply $input)
echo "The result is $result"
done
```
这个脚本首先定义了一个名为`multiply`的函数来实现累乘功能,它接受任意数量的参数,将它们相乘并返回结果。然后程序进入一个无限循环,读取用户输入的数字列表,如果输入为"q"则退出循环。每次输入数字列表后,程序调用`multiply`函数计算结果,并输出累乘结果。
编写一个shell程序可以完成以下执行过程。 [root@localhost ~]# ./main.sh Input a list of number: 1 2 3 4 5 6 the result is 21 Input a list of number: 1 1 1 the result is 3 Input a list of number: q [root@localhost ~]#
以下是一个实现上述功能的 Shell 脚本:
```shell
#!/bin/bash
while true; do
read -p "Input a list of number: " input
sum=0
for i in $input; do
if ! echo $i | grep -qE "^[0-9]+$"; then
echo "Invalid input, please input numbers only"
continue 2
fi
sum=$((sum + i))
done
echo "the result is $sum"
done
```
说明:
1. 使用 while 循环不断提示用户输入数列,直到用户输入 q 退出程序;
2. 使用 read 命令读取用户输入的数列,并将其保存到变量 input 中;
3. 使用 for 循环遍历数列中的每个元素,使用 grep 命令判断每个元素是否为数字;
4. 如果元素不是数字,则输出提示信息并跳过当前循环;
5. 如果元素是数字,则将其加入到变量 sum 中;
6. 循环结束后,输出变量 sum 的值。
阅读全文
相关推荐
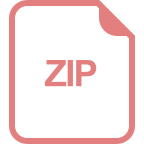
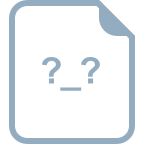








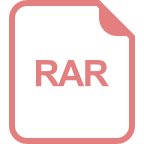




