vue3的ts封装请求具体代码
时间: 2023-12-01 14:05:10 浏览: 66
Sure, I can answer that! Here's an example of how to use TypeScript to encapsulate an API request in Vue 3:
```typescript
import axios, { AxiosRequestConfig, AxiosResponse } from 'axios'
import { ref } from 'vue'
interface ApiResponse<T> {
code: number
data: T
message: string
}
export function useApi<T>(url: string, config?: AxiosRequestConfig) {
const loading = ref(false)
const error = ref('')
const data = ref<T | null>(null)
const fetchData = async () => {
loading.value = true
try {
const response: AxiosResponse<ApiResponse<T>> = await axios.get(url, config)
if (response.data.code === 0) {
data.value = response.data.data
} else {
error.value = response.data.message
}
} catch (e) {
error.value = 'Network Error'
}
loading.value = false
}
return {
loading,
error,
data,
fetchData,
}
}
```
This function defines a generic function `useApi` that takes a `url` and an optional `config` object for Axios requests. The function returns an object with `loading`, `error`, `data`, and `fetchData` properties. The `fetchData` method sends a GET request to the specified URL and updates the `loading`, `error`, and `data` properties accordingly.
To use this function in a Vue component, you would import it and call it like this:
```vue
<template>
<div>
<p v-if="loading">Loading...</p>
<p v-if="error">{{ error }}</p>
<p v-if="data">{{ data }}</p>
<button @click="fetchData">Fetch Data</button>
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue'
import { useApi } from './api' // assuming the above code is in a separate file
export default defineComponent({
setup() {
const { loading, error, data, fetchData } = useApi<string>('/api/endpoint')
return {
loading,
error,
data,
fetchData,
}
},
})
</script>
```
In this example, the component calls `useApi('/api/endpoint')` to create a reactive `loading`, `error`, `data`, and `fetchData` state. The `data` state is assumed to be a string, but could be any type specified when calling the `useApi` function. The component template conditionally renders the loading indicator, error message, and data text depending on the values of the reactive state. The `fetchData` method is called when the user clicks the button, triggering the API request and updating the reactive state accordingly.
阅读全文
相关推荐
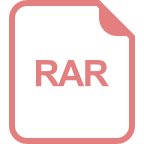















