vue3 ts接口请求
时间: 2023-10-09 14:15:59 浏览: 132
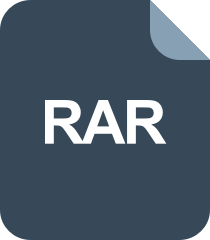
vue3+TS+axios+route+element plus 可二次开发的模板,包括请求使用方法,完美封装 上手即用

在 Vue 3 中使用 TypeScript 进行接口请求,你可以按照以下步骤进行操作:
1. 安装依赖:首先,你需要安装 Axios 库来进行接口请求。在项目根目录下运行以下命令:
```bash
npm install axios
```
2. 创建接口定义:在你的 TypeScript 文件中创建一个接口来定义返回数据的结构。例如,创建一个 `User` 接口来定义用户信息:
```typescript
interface User {
id: number;
name: string;
email: string;
}
```
3. 创建服务文件:创建一个服务文件来封装接口请求逻辑。在该文件中,你可以使用 Axios 来发送 HTTP 请求,并使用 TypeScript 的泛型来指定响应的数据类型。例如,创建一个 `UserService` 文件:
```typescript
import axios, { AxiosResponse } from 'axios';
const API_BASE_URL = 'https://api.example.com';
export async function getUserById(id: number): Promise<User> {
const response: AxiosResponse<User> = await axios.get(`${API_BASE_URL}/users/${id}`);
return response.data;
}
```
4. 在组件中使用服务:在你的 Vue 组件中导入并使用服务来进行接口请求。例如,在一个 `UserComponent` 组件中使用 `UserService` 的 `getUserById` 方法:
```typescript
<script lang="ts">
import { defineComponent, ref } from 'vue';
import { getUserById } from './services/UserService';
export default defineComponent({
name: 'UserComponent',
setup() {
const user = ref<User | null>(null);
const fetchUser = async (id: number) => {
user.value = await getUserById(id);
};
// 使用 fetchUser 方法来获取用户信息
fetchUser(1);
return {
user,
};
},
});
</script>
```
这样,你就可以在 Vue 3 中使用 TypeScript 进行接口请求了。记得根据你的项目需求进行相应的调整。希望能对你有所帮助!如果还有任何问题,请随时提问。
阅读全文
相关推荐
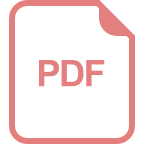















