帮我写一个对二维数组进行均值滤波的函数
时间: 2024-12-28 12:32:17 浏览: 0
当然,二维数组的均值滤波是一种常见的图像处理技术,它的目的是通过计算周围像素的平均值来平滑图像,减少噪声的影响。以下是一个简单的C++函数,使用5x5的邻域进行均值滤波:
```cpp
#include <vector>
// 均值滤波器模板函数,接受一个二维整数数组(矩阵)和大小为n*n的滤波窗口
template<int n>
std::vector<std::vector<int>> meanFilter(const std::vector<std::vector<int>>& inputArray) {
const int width = inputArray.size();
const int height = inputArray[0].size();
// 创建新的滤波结果数组
std::vector<std::vector<int>> filteredArray(width, std::vector<int>(height, 0));
for (int i = n / 2; i < width - n / 2; ++i) {
for (int j = n / 2; j < height - n / 2; ++j) {
int sum = 0;
for (int x = i - n / 2; x <= i + n / 2; ++x) {
if (x >= 0 && x < width) {
for (int y = j - n / 2; y <= j + n / 2; ++y) {
if (y >= 0 && y < height) {
sum += inputArray[x][y];
}
}
}
}
filteredArray[i][j] = sum / (n * n);
}
}
return filteredArray;
}
// 使用示例
int main() {
std::vector<std::vector<int>> input = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
std::vector<std::vector<int>> filtered = meanFilter<5>(input);
// 打印滤波后的结果
for (const auto& row : filtered) {
for (int val : row) {
std::cout << val << " ";
}
std::cout << "\n";
}
return 0;
}
```
这个函数`meanFilter`接收一个二维整数数组`inputArray`,并返回一个新的二维数组,其中每个元素都是原数组对应位置周围n*n个像素的平均值。
阅读全文
相关推荐
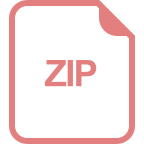
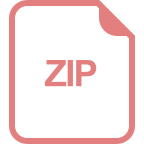
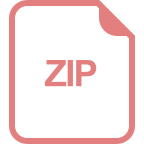




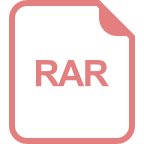
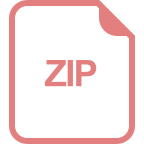
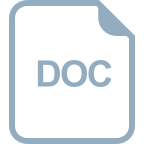





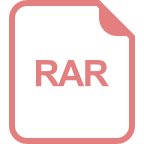
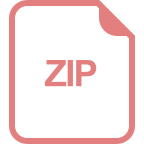
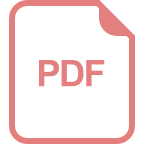
