cv2.findContours(mark, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
时间: 2024-10-04 07:03:34 浏览: 35
`cv2.findContours()` 函数是OpenCV库中的一个用于查找轮廓的方法,它在图像处理中非常有用。这个函数接收两个参数:输入图像(这里是`edged.copy()`)和一些标志来定义搜索方式。
`cv2.RETR_EXTERNAL`标志表示只寻找外部轮廓,即最外层的对象边缘。这意味着函数不会跟踪内部孔洞或物体的一部分,仅返回与背景分离的完整形状。
`cv2.CHAIN_APPROX_SIMPLE`标志则控制结果轮廓的存储方式。`CHAIN_APPROX_SIMPLE`会压缩垂直和水平连续像素点,只保留终点坐标,这对于简化轮廓并节省内存空间很有帮助。
示例演示:
```python
# 假设`edged`是经过边缘检测处理后的二值图像
cnts = cv2.findContours(edged.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
if cnts and len(cnts[0]) > 0: # 如果找到轮廓
for contour in cnts[0]:
x, y, w, h = cv2.boundingRect(contour)
print(f"Contour at ({x}, {y}) with dimensions ({w}, {h})")
else:
print("No contours found.")
```
这段代码会打印每个外部轮廓的位置及其边界框尺寸。
相关问题
from skimage.segmentation import slic, mark_boundaries import torchvision.transforms as transforms import numpy as np from PIL import Image import matplotlib.pyplot as plt import cv2 # 加载图像 image = Image.open('img.png') # 转换为 PyTorch 张量 transform = transforms.ToTensor() img_tensor = transform(image).unsqueeze(0) # 将 PyTorch 张量转换为 Numpy 数组 img_np = img_tensor.numpy().transpose(0, 2, 3, 1)[0] # 使用 SLIC 算法生成超像素标记图 segments = slic(img_np, n_segments=100, compactness=10) # 可视化超像素标记图 segment_img = mark_boundaries(img_np, segments) # 将 Numpy 数组转换为 PIL 图像 segment_img = Image.fromarray((segment_img * 255).astype(np.uint8)) # 保存超像素标记图 segment_img.save('segments.jpg') n_segments = np.max(segments) + 1 # 初始化超像素块的区域 segment_regions = np.zeros((n_segments, img_np.shape[0], img_np.shape[1])) # 遍历每个超像素块 for i in range(n_segments): # 获取当前超像素块的掩码 mask = (segments == i) # 将当前超像素块的掩码赋值给超像素块的区域 segment_regions[i][mask] = 1 # 保存超像素块的区域 np.save('segment_regions.npy', segment_regions) # 加载超像素块的区域 segment_regions = np.load('segment_regions.npy') # 取出第一个超像素块的区域 segment_region = segment_regions[0] segment_region = (segment_region * 255).astype(np.uint8) # 显示超像素块的区域 plt.imshow(segment_region, cmap='gray') plt.show(),将上述显示的超像素块区域的边缘添加一种颜色
我已经回答过这个问题了,你可以参考上一个回答。以下是修改后的代码示例:
```
# 找到超像素块区域的轮廓
contours, _ = cv2.findContours(segment_region, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 将轮廓绘制在超像素块区域上,并设置颜色和线条宽度
segment_region_with_contours = cv2.drawContours(segment_region, contours, -1, (255, 0, 0), 2)
# 显示带有边缘颜色的超像素块区域
plt.imshow(segment_region_with_contours, cmap='gray')
plt.show()
```
其中,`cv2.RETR_EXTERNAL` 表示只寻找最外层轮廓,`cv2.CHAIN_APPROX_SIMPLE` 表示只保留轮廓的端点。`-1` 表示绘制所有轮廓。`(255, 0, 0)` 表示边缘颜色为蓝色,`2` 表示线条宽度为 2 个像素。
from skimage.segmentation import slic, mark_boundaries import torchvision.transforms as transforms import numpy as np from PIL import Image import matplotlib.pyplot as plt # 加载图像 image = Image.open('img.png') # 转换为 PyTorch 张量 transform = transforms.ToTensor() img_tensor = transform(image).unsqueeze(0) # 将 PyTorch 张量转换为 Numpy 数组 img_np = img_tensor.numpy().transpose(0, 2, 3, 1)[0] # 使用 SLIC 算法生成超像素标记图 segments = slic(img_np, n_segments=100, compactness=10) # 可视化超像素标记图 segment_img = mark_boundaries(img_np, segments) # 将 Numpy 数组转换为 PIL 图像 segment_img = Image.fromarray((segment_img * 255).astype(np.uint8)) # 保存超像素标记图 segment_img.save('segments.jpg') n_segments = np.max(segments) + 1 # 初始化超像素块的区域 segment_regions = np.zeros((n_segments, img_np.shape[0], img_np.shape[1])) # 遍历每个超像素块 for i in range(n_segments): # 获取当前超像素块的掩码 mask = (segments == i) # 将当前超像素块的掩码赋值给超像素块的区域 segment_regions[i][mask] = 1 # 保存超像素块的区域 np.save('segment_regions.npy', segment_regions) # 加载超像素块的区域 segment_regions = np.load('segment_regions.npy') # 取出第一个超像素块的区域 segment_region = segment_regions[37] segment_region = (segment_region * 255).astype(np.uint8) # 显示超像素块的区域 plt.imshow(segment_region, cmap='gray') plt.show(),将上述超像素块的区域汇总到一张图上,每块边缘用黄线表示
可以使用 `cv2.drawContours` 函数来绘制边缘,具体代码如下:
```python
import cv2
# 初始化空白图像
output = np.zeros_like(img_np)
# 遍历每个超像素块
for i in range(n_segments):
# 获取当前超像素块的掩码
mask = segments == i
# 将当前超像素块的掩码赋值给输出图像
output[mask] = segment_regions[i] * 255
# 绘制超像素块的边缘
contours, _ = cv2.findContours(mask.astype(np.uint8), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(output, contours, -1, (255, 255, 0), 1)
# 显示超像素块的区域和边缘
plt.imshow(output)
plt.show()
```
上述代码中,`cv2.findContours` 函数用于寻找超像素块的轮廓,`cv2.drawContours` 函数用于绘制轮廓。绘制轮廓时,我们使用了黄色 `(255, 255, 0)` 的线条,并设置线条宽度为 1 像素。
阅读全文
相关推荐
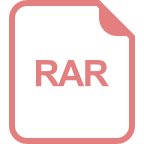
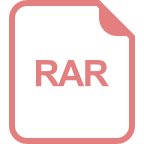
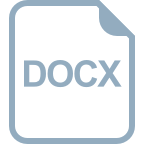
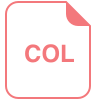

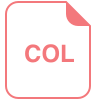
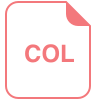
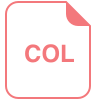
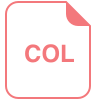
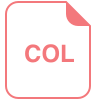
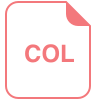
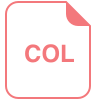

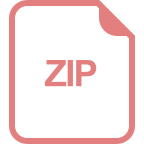
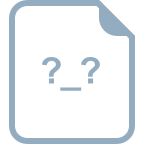