def build_model(max_features, maxlen): """Build LSTM model""" model = Sequential() model.add(Embedding(max_features, 128, input_length=maxlen)) model.add(LSTM(128)) model.add(Dropout(0.5)) model.add(Dense(1)) model.add(Activation('sigmoid')) # model.add(tf.keras.layers.BatchNormalization()) model.compile(loss='binary_crossentropy', optimizer='rmsprop') return model这个模型重写 get_config()
时间: 2024-03-06 15:51:26 浏览: 233
好的,以下是重新实现的模型代码,其中包括了重写 `get_config()` 方法:
```python
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Embedding, LSTM, Dropout, Dense, Activation
class LSTMModel(Sequential):
def __init__(self, max_features, maxlen, **kwargs):
super(LSTMModel, self).__init__(**kwargs)
self.max_features = max_features
self.maxlen = maxlen
self.add(Embedding(max_features, 128, input_length=maxlen))
self.add(LSTM(128))
self.add(Dropout(0.5))
self.add(Dense(1))
self.add(Activation('sigmoid'))
self.compile(loss='binary_crossentropy', optimizer='rmsprop')
def get_config(self):
config = super(LSTMModel, self).get_config()
config.update({'max_features': self.max_features,
'maxlen': self.maxlen})
return config
```
在这个例子中,我们继承了 `Sequential` 类,并实现了 `__init__()` 方法和 `get_config()` 方法。在 `__init__()` 方法中,我们按照原始模型的结构构建了模型,并编译了模型。在 `get_config()` 方法中,我们返回了一个字典,其中包含了模型的配置信息。
需要注意的是,因为 `Sequential` 类已经实现了 `get_config()` 方法,所以我们可以直接调用父类的 `get_config()` 方法,并将自定义的配置信息添加到返回的字典中。
希望这个回答能够解决你的问题。
阅读全文
相关推荐
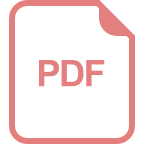
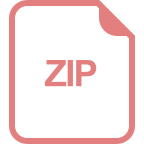
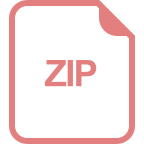








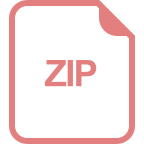
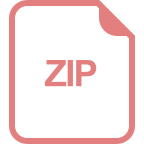
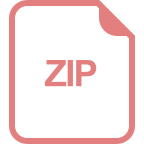
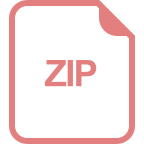
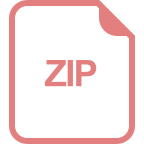