import matplotlib.pyplot as plt import tensorflow as tf from tensorflow import keras import numpy as np #加载IMDB数据 imdb = keras.datasets.imdb (train_data, train_labels), (test_data, test_labels) = imdb.load_data(num_words=100) print("训练记录数量:{},标签数量:{}".format(len(train_data),len(train_labels))) print(train_data[0]) #数据标准化 train_data = keras.preprocessing.sequence.pad_sequences(train_data,value=0,padding='post',maxlen=256) text_data = keras.preprocessing.sequence.pad_sequences(train_data,value=0,padding='post',maxlen=256) print(train_data[0]) #构建模型 vocab_size = 10000 model = tf.keras.Sequential([tf.keras.layers.Embedding(vocab_size, 64), tf.keras.layers.Bidirectional(tf.keras.layers.LSTM(64)), tf.keras.layers.Dense(64,activation='relu'), tf.keras.layers.Dense(1) ]) model.summary() #配置并训练模型 model.compile(optimizer='adam',loss='binary_crossentropy',metrics=['accuracy']) x_val = train_data[:10000] partial_x_train = train_data[10000:] y_val = train_labels[:10000] partial_y_train = train_labels[10000:] history = model.fit(partial_x_train,partial_y_train,epochs=1,batch_size=512,validation_data=(x_val,y_val),verbose=1) #测试性能 results = model.evaluate(test_data, test_labels, verbose=2) print(results) #训练过程可视化 history_dict = history.history print(history_dict.keys()) def plot_graphs(history, string): plt.plot(history.history[string]) plt.plot(history.history['val_'+string]) plt.xlabel("Epochs") plt.ylabel(string) plt.legend([string,'val_'+string]) plt.show() plot_graphs(history,"accuracy") plot_graphs(history,"loss")
时间: 2024-03-14 15:49:07 浏览: 114
这段代码是一个使用IMDB数据集进行情感分析的模型。该模型使用了嵌入层、双向LSTM层和两个全连接层。以下是这段代码的功能和步骤:
1. 加载 IMDB 数据集,包括训练数据和测试数据。
2. 对训练数据进行标准化,使用 `keras.preprocessing.sequence.pad_sequences` 来将序列填充或截断到相同的长度。
3. 构建模型,包括一个嵌入层、一个双向LSTM层和两个全连接层。
4. 配置并训练模型,使用 `model.compile` 来配置模型的优化器、损失函数和评价指标,然后使用 `model.fit` 来训练模型。
5. 测试模型性能,使用 `model.evaluate` 来评估模型在测试数据上的性能。
6. 可视化训练过程,使用 `plot_graphs` 函数来绘制模型在训练和验证集上的损失和准确率。
需要注意的是,这段代码中 `text_data` 的赋值是错误的,应该是 `text_data = keras.preprocessing.sequence.pad_sequences(test_data,value=0,padding='post',maxlen=256)`。同时,代码中只训练了一次,因为 `epochs=1`。如果想要更好的模型性能,应该增加训练次数。
相关问题
import tensorflow as tf import numpy as np import matplotlib.pyplot as plt import pandas as pd from sklearn.utils import shuffle from sklearn.preprocessing import scale df = pd.read_csv("C:\\boston.csv",header=0) ds = df.values from sklearn.datasets import load_boston boston = load_boston() X = boston.data y = boston.target from sklearn.model_selection import train_test_split X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) from sklearn.preprocessing import StandardScaler scaler = StandardScaler() X_train = scaler.fit_transform(X_train) X_test = scaler.transform(X_test) from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense model = Sequential([ Dense(64, activation='relu', input_shape=(X_train.shape[1],)), Dense(64, activation='relu'), Dense(64, activation='relu'), Dense(1) ]) model.compile(loss='mean_squared_error', optimizer='adam') history = model.fit(X_train, y_train, validation_split=0.1, epochs=100, batch_size=32) from sklearn.metrics import mean_squared_error y_pred = model.predict(x_test)mse = mean_squared_error(y_test, y_pred)print('MSE:’, mse) import matplotlib.pyplot as plt plt.plot(history.history['accuracy'], label='train') plt.plot(history.history['val_accuracy'], label='validation') plt.legend() plt.show()
这段代码使用TensorFlow和Keras实现了一个神经网络模型,用于预测波士顿房价。以下是代码的解释:
首先,导入必要的库和模块:
```
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
from sklearn.utils import shuffle
from sklearn.preprocessing import scale
```
然后,读取波士顿房价数据集并对其进行预处理:
```
df = pd.read_csv("C:\\boston.csv",header=0)
ds = df.values
```
接着,从sklearn.datasets模块中加载波士顿房价数据集,并将其分为训练集和测试集:
```
from sklearn.datasets import load_boston
boston = load_boston()
X = boston.data
y = boston.target
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
对训练集和测试集进行标准化处理:
```
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
```
定义一个包含4个Dense层的神经网络模型:
```
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
model = Sequential([
Dense(64, activation='relu', input_shape=(X_train.shape[1],)),
Dense(64, activation='relu'),
Dense(64, activation='relu'),
Dense(1)
])
```
编译模型并训练:
```
model.compile(loss='mean_squared_error', optimizer='adam')
history = model.fit(X_train, y_train, validation_split=0.1, epochs=100, batch_size=32)
```
使用模型对测试集进行预测,并计算均方误差:
```
from sklearn.metrics import mean_squared_error
y_pred = model.predict(x_test)
mse = mean_squared_error(y_test, y_pred)
print('MSE:’, mse)
```
最后,绘制模型的训练和验证准确率曲线:
```
import matplotlib.pyplot as plt
plt.plot(history.history['accuracy'], label='train')
plt.plot(history.history['val_accuracy'], label='validation')
plt.legend()
plt.show()
```
import numpy as np import matplotlib.pyplot as plt from sklearn.datasets import make_classification import tensorflow as tf from keras.models import Sequential from keras.layers import Dense # 使用 sklearn 的 make_classification 方法生成随机的二维数据 X, y = make_classification(n_samples=500, n_features=2, n_informative=2, n_redundant=0, n_classes=2, random_state=1) # 使用 matplotlib 绘制生成的二维数据 plt.scatter(X[:, 0], X[:, 1], marker='o', c=y, s=25, edgecolor='k') plt.show() # 定义两层神经网络模型 model = Sequential() model.add(Dense(2, input_dim=2, activation='relu')) model.add(Dense(1, activation='sigmoid')) # 编译模型 model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy']) # 训练模型 model.fit(X, y, epochs=50, batch_size=10) # 评估模型 _, accuracy = model.evaluate(X, y) print('Accuracy: %.2f' % (accuracy*100))详细解释一下
这段代码是使用Keras和TensorFlow库来构建一个简单的二分类神经网络模型。以下是代码的详细解释:
1. 导入所需的库:导入 numpy、matplotlib.pyplot、make_classification 方法从 sklearn.datasets 中、tensorflow 和 keras 中的 Sequential 和 Dense。
2. 使用 make_classification 方法生成随机的二维数据:这个方法可以生成具有指定特征数量和类别数量的随机数据。在这个例子中,我们生成了500个样本,每个样本有2个特征,并且有2个信息特征和0个冗余特征。
3. 使用 matplotlib 绘制生成的二维数据:使用 scatter 函数绘制散点图,X[:, 0] 和 X[:, 1] 是样本数据的两个特征,c=y 表示根据标签 y 进行着色,s=25 设置散点的大小为25,edgecolor='k' 设置散点的边框颜色为黑色。
4. 定义两层神经网络模型:创建一个 Sequential 模型,并添加两个 Dense 层。第一个 Dense 层有2个神经元,输入维度为2,激活函数为 ReLU;第二个 Dense 层有1个神经元,激活函数为 Sigmoid。
5. 编译模型:使用 compile 方法来配置模型的损失函数、优化器和评估指标。在这个例子中,损失函数为 binary_crossentropy(二分类交叉熵),优化器为 Adam,评估指标为准确率。
6. 训练模型:使用 fit 方法来训练模型。传入训练数据 X 和标签 y,设定训练的轮数为50,批次大小为10。
7. 评估模型:使用 evaluate 方法来评估模型在训练数据上的性能。将训练数据 X 和标签 y 作为参数传入,返回损失值和准确率。最后打印出准确率的百分比。
希望这样的解释对你有帮助!如果你还有其他问题,请随时提问。
阅读全文
相关推荐
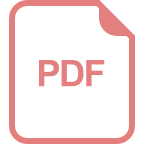
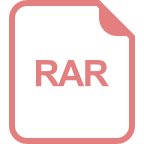
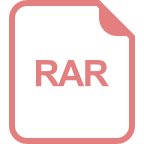








