import numpy import tensorflow as tf import matplotlib.pyplot as plt fashion =tf.keras.datasets.fashion_mnist (x_train,y_train),(x_test,y_test)=fashion.load_data() plt.figure(figsize=(30,30)) for i in range(30): plt.subplot(3,10,i+1) #print("第一张图片****************") #print(x_train[0]) #print("第一张图片对应的数字******************") #print(y_train[0]) plt.imshow(x_train[i],cmap=plt.cm.gray_r) #plt.show() model=tf.keras.models.Sequential([ #把二维数据转成一位数据 tf.keras.layers.Flatten(input_shape=(28,28)), #定义神经网络全连接层,参数是神经元个数和使用的激活函数 tf.keras.layers.Dense(128,activation='relu'), #设置遗忘率 tf.keras.layers.Dropout(0.2), #定义输出(输出10种类型,softmax实现分类的概率分布 tf.keras.layers.Dense(10,activation='softmax') ]) #优化模型 model.compile(#选择优化器 optimizer='adam', #定义损失函数 loss='sparse_categorical_crossentropy', #模型的评估 metrics=['accuracy']) #训练模型(训练集和次数) model.fit(x_train,y_train,epochs=5) #验证模型(测试集) model.evaluate(x_test,y_test) #测试集测试 class_names=['上衣','裤子','套头衫','连衣裙','外套','凉鞋','衬衫','运动鞋','包包','短靴'] imgDate=(numpy.expand_dims(x_test[0],0)) predictions_single=model.predict(imgDate) max=numpy.argmax(predictions_single) print(class_names[max]) plt.imshow(x_test[0],cmap=plt.cm.gray_r) plt.show()
时间: 2024-01-24 15:03:41 浏览: 31
这段代码是使用Fashion-MNIST数据集训练一个基本的神经网络模型,并使用该模型进行图像分类。如果你想将其转变为图像人脸识别,你需要进行以下步骤:
1. 首先,你需要收集人脸图像数据集。你可以使用已有的人脸数据集,如LFW、CelebA或CASIA等,或者自己收集数据集。
2. 对于人脸图像数据集,你需要进行数据预处理和增强,以提高模型的性能。例如,你可以使用OpenCV或PIL库对图像进行裁剪、缩放、旋转、翻转等操作,以及使用数据增强技术,如随机裁剪、旋转、亮度调整等。
3. 接下来,你需要使用卷积神经网络(CNN)来训练你的人脸识别模型。你可以使用Keras或PyTorch等深度学习框架来实现。
4. 在训练模型之前,你需要将数据集分成训练集、验证集和测试集。训练集用于模型的训练,验证集用于模型的调优,测试集用于评估模型的性能。
5. 接着,你需要定义一个CNN模型,并将其编译。例如,你可以使用多个卷积层和池化层,以及全连接层和输出层。
6. 然后,你需要使用训练集来训练模型,并使用验证集来调整模型的超参数。当模型在验证集上的性能不再提高时,就可以停止训练。
7. 最后,你可以使用测试集来评估模型的性能,并进行人脸识别。
需要注意的是,人脸识别是一个复杂的任务,需要深入了解相关知识和技术才能进行。此处提供的步骤只是一个大致的指南,具体实现还需要根据你的具体情况进行调整。
相关问题
下面的代码哪里有问题,帮我改一下from __future__ import print_function import numpy as np import tensorflow import keras from keras.models import Sequential from keras.layers import Dense,Dropout,Flatten from keras.layers import Conv2D,MaxPooling2D from keras import backend as K import tensorflow as tf import datetime import os np.random.seed(0) from sklearn.model_selection import train_test_split from PIL import Image import matplotlib.pyplot as plt from keras.datasets import mnist images = [] labels = [] (x_train,y_train),(x_test,y_test)=mnist.load_data() X = np.array(images) print (X.shape) y = np.array(list(map(int, labels))) print (y.shape) x_train, x_test, y_train, y_test = train_test_split(X, y, test_size=0.30, random_state=0) print (x_train.shape) print (x_test.shape) print (y_train.shape) print (y_test.shape) ############################ ########## batch_size = 20 num_classes = 4 learning_rate = 0.0001 epochs = 10 img_rows,img_cols = 32 , 32 if K.image_data_format() =='channels_first': x_train =x_train.reshape(x_train.shape[0],1,img_rows,img_cols) x_test = x_test.reshape(x_test.shape[0],1,img_rows,img_cols) input_shape = (1,img_rows,img_cols) else: x_train = x_train.reshape(x_train.shape[0],img_rows,img_cols,1) x_test = x_test.reshape(x_test.shape[0],img_rows,img_cols,1) input_shape =(img_rows,img_cols,1) x_train =x_train.astype('float32') x_test = x_test.astype('float32') x_train /= 255 x_test /= 255 print('x_train shape:',x_train.shape) print(x_train.shape[0],'train samples') print(x_test.shape[0],'test samples')
代码中存在以下问题:
1. 变量 images 和 labels 没有被赋值,而后面却使用了它们来构造 X 和 y,导致 X 和 y 的值都为空。
2. 导入的 mnist 数据集没有被使用,而是使用了未定义的 images 和 labels,需要将其替换为 mnist 数据集。
3. input_shape 的值应该是三元组 (img_rows, img_cols, 1),而代码中缺少了最后一个 1。
4. 在 if K.image_data_format() =='channels_first': 分支中,x_train 和 x_test 被改变了形状,但 y_train 和 y_test 却没有被改变,需要将其同步修改。
下面是修改后的代码:
```
from __future__ import print_function
import numpy as np
import tensorflow
import keras
from keras.models import Sequential
from keras.layers import Dense, Dropout, Flatten
from keras.layers import Conv2D, MaxPooling2D
from keras import backend as K
import tensorflow as tf
import datetime
import os
np.random.seed(0)
from sklearn.model_selection import train_test_split
from PIL import Image
import matplotlib.pyplot as plt
from keras.datasets import mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
print(x_train.shape)
print(y_train.shape)
############################
##########
batch_size = 20
num_classes = 10
learning_rate = 0.0001
epochs = 10
img_rows, img_cols = 32, 32
if K.image_data_format() == 'channels_first':
x_train = x_train.reshape(x_train.shape[0], 1, img_rows, img_cols)
x_test = x_test.reshape(x_test.shape[0], 1, img_rows, img_cols)
input_shape = (img_rows, img_cols, 1)
else:
x_train = x_train.reshape(x_train.shape[0], img_rows, img_cols, 1)
x_test = x_test.reshape(x_test.shape[0], img_rows, img_cols, 1)
input_shape = (img_rows, img_cols, 1)
y_train = keras.utils.to_categorical(y_train, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
x_train = x_train.astype('float32')
x_test = x_test.astype('float32')
x_train /= 255
x_test /= 255
print('x_train shape:', x_train.shape)
print(x_train.shape[0], 'train samples')
print(x_test.shape[0], 'test samples')
print('input_shape:', input_shape)
```
import numpy as np import tensorflow as tf from tensorflow import keras import matplotlib.pyplot as plt Let us define a plt function for simplicity def plt_loss(x,training_metric,testing_metric,ax,colors = ['b']): ax.plot(x,training_metric,'b',label = 'Train') ax.plot(x,testing_metric,'k',label = 'Test') ax.set_xlabel('Epochs') ax.set_ylabel('Accuracy') plt.legend() plt.grid() plt.show() tf.keras.utils.set_random_seed(1) We import the Minist Dataset using Keras.datasets (train_data, train_labels), (test_data, test_labels) = keras.datasets.mnist.load_data() We first vectorize the image (28*28) into a vector (784) train_data = train_data.reshape(train_data.shape[0],train_data.shape[1]train_data.shape[2]) # 60000784 test_data = test_data.reshape(test_data.shape[0],test_data.shape[1]test_data.shape[2]) # 10000784 We next change label number to a 10 dimensional vector, e.g., 1-> train_labels = keras.utils.to_categorical(train_labels,10) test_labels = keras.utils.to_categorical(test_labels,10) start to build a MLP model N_batch_size = 5000 N_epochs = 100 lr = 0.01 we build a three layer model, 784 -> 64 -> 10 MLP_3 = keras.models.Sequential([ keras.layers.Dense(128, input_shape=(784,),activation='relu'), keras.layers.Dense(64, activation='relu'), keras.layers.Dense(10,activation='softmax') ]) MLP_3.compile( optimizer=keras.optimizers.Adam(lr), loss= 'categorical_crossentropy', metrics = ['accuracy'] ) History = MLP_3.fit(train_data,train_labels, batch_size = N_batch_size, epochs = N_epochs,validation_data=(test_data,test_labels), shuffle=False) train_acc = History.history['accuracy'] test_acc = History.history对于该模型,使用不同数量的训练数据(5000,10000,15000,…,60000,公差=5000的等差数列),绘制训练集和测试集准确率(纵轴)关于训练数据大小(横轴)的曲线
Sure, here's the code:
```
import numpy as np
import tensorflow as tf
from tensorflow import keras
import matplotlib.pyplot as plt
# Define a plt function for simplicity
def plt_loss(x,training_metric,testing_metric,ax,colors = ['b']):
ax.plot(x,training_metric,'b',label = 'Train')
ax.plot(x,testing_metric,'k',label = 'Test')
ax.set_xlabel('Epochs')
ax.set_ylabel('Accuracy')
plt.legend()
plt.grid()
plt.show()
tf.keras.utils.set_random_seed(1)
# Import the MNIST Dataset using Keras.datasets
(train_data, train_labels), (test_data, test_labels) = keras.datasets.mnist.load_data()
# Vectorize the image (28*28) into a vector (784)
train_data = train_data.reshape(train_data.shape[0],train_data.shape[1]*train_data.shape[2]) # 60000*784
test_data = test_data.reshape(test_data.shape[0],test_data.shape[1]*test_data.shape[2]) # 10000*784
# Change label number to a 10 dimensional vector, e.g., 1-> [0,1,0,0,0,0,0,0,0,0]
train_labels = keras.utils.to_categorical(train_labels,10)
test_labels = keras.utils.to_categorical(test_labels,10)
# Start to build a MLP model
N_batch_size = 5000
N_epochs = 100
lr = 0.01
# Build a three layer model, 784 -> 64 -> 10
MLP_3 = keras.models.Sequential([
keras.layers.Dense(128, input_shape=(784,),activation='relu'),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(10,activation='softmax')
])
MLP_3.compile(
optimizer=keras.optimizers.Adam(lr),
loss= 'categorical_crossentropy',
metrics = ['accuracy']
)
# Store the training history
train_acc = []
test_acc = []
for i in range(5000,65000,5000):
print('Training on',i,'samples')
History = MLP_3.fit(train_data[:i],train_labels[:i], batch_size = N_batch_size, epochs = N_epochs,validation_data=(test_data,test_labels), shuffle=False)
train_acc.append(History.history['accuracy'][-1])
test_acc.append(History.history['val_accuracy'][-1])
# Plot the training and testing accuracy as a function of training data size
plt.figure(figsize=(8,5))
plt.plot(range(5000,65000,5000),train_acc,'b',label = 'Train')
plt.plot(range(5000,65000,5000),test_acc,'k',label = 'Test')
plt.xlabel('Training Data Size')
plt.ylabel('Accuracy')
plt.legend()
plt.grid()
plt.show()
```
This code trains a three-layer MLP model on the MNIST dataset using Keras. It then loops through different numbers of training samples (5000, 10000, 15000, ..., 60000) and trains the model on each subset of the data for 100 epochs. After each training run, it records the final training and testing accuracy. Finally, it plots a graph of accuracy as a function of training data size.
相关推荐
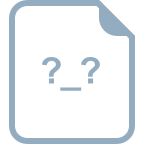
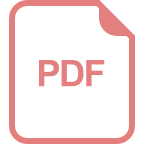
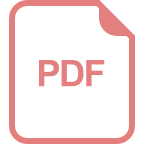












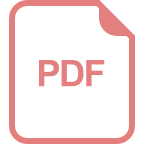