opencv批量读取图片python函数形式
时间: 2023-06-18 10:02:40 浏览: 357
你可以使用OpenCV库中的cv2.imread()函数来读取图片。以下是一个示例函数,它可以批量读取指定路径下的所有图片:
```python
import cv2
import os
def read_images_from_folder(folder_path):
images = []
for filename in os.listdir(folder_path):
img = cv2.imread(os.path.join(folder_path,filename))
if img is not None:
images.append(img)
return images
```
这个函数接受一个文件夹路径作为参数,遍历文件夹中的所有文件,如果是图片文件就读取并添加到一个列表中,最后返回这个列表。
相关问题
opencv批量处理图片python
使用OpenCV和Python可以批量处理图片,以下是一个简单的例子:
首先,您需要导入OpenCV和Python的os库,以便处理文件夹中的图像文件。然后,您可以使用os.listdir()函数列出目录中所有的图像文件,然后使用cv2.imread()函数读取每个文件并进行所需的处理,例如调整大小,转换颜色空间,应用过滤器等。最后,您可以使用cv2.imwrite()函数将处理后的图像保存到另一个目录中。
以下是一个基本的代码框架:
```python
import cv2
import os
# 设置要处理的图像文件夹路径
input_folder = "input_folder"
output_folder = "output_folder"
# 循环处理每个图像文件
for filename in os.listdir(input_folder):
# 读取图像
img = cv2.imread(os.path.join(input_folder, filename))
# 进行所需的处理
# 保存处理后的图像
cv2.imwrite(os.path.join(output_folder, filename), img)
```
当然,您可以根据需要添加其他功能和处理步骤。
python OpenCV 批量读取图像
您可以使用以下代码批量读取图像:
```python
import cv2
import os
def batch_read_images(folder_path):
images = []
for filename in os.listdir(folder_path):
file_path = os.path.join(folder_path, filename)
if os.path.isfile(file_path):
image = cv2.imread(file_path)
if image is not None:
images.append(image)
return images
# 指定图像文件夹的路径
folder_path = 'path/to/your/images/folder'
# 调用函数批量读取图像
images = batch_read_images(folder_path)
# 遍历显示读取的图像
for image in images:
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
您需要将 `folder_path` 替换为实际存放图像文件的文件夹路径。上述代码会遍历指定文件夹中的所有文件,读取图像文件并将其存储在一个列表中。然后,您可以使用 OpenCV 的 `imshow` 函数显示每个图像。请确保在显示完图像后使用 `destroyAllWindows` 函数关闭窗口。
希望能对您有所帮助!如果有任何疑问,请随时提问。
阅读全文
相关推荐
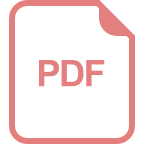
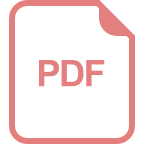





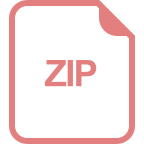
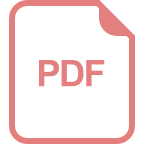





