下一步,在“WEB-INF/pages/course”目录中,创建add_course_basic.jsp文件,设计表单,提交课程信息。课程信息包括课程编号(cid),课程名称(cname),学时数(period),教材名称(bname),出版社(pub),价格(price)。下一步,在“WEB-INF/pages/course”目录中,创建course_info_basic.jsp文件,以EL方式显示请求域中的对象。下一步,在com.javaee.ex12.po包中创建实体类Course,要求属性与表单元素的name属性一致。下一步,在控制器类CourseController中,添加处理方法toCourseAddPojo和CourseAddPojo。在CourseAddPojo方法中,以POJO方式绑定数据。下一步,在“WEB-INF/pages/course”目录中,创建add_course_pojo.jsp文件,将add_course_basic.jsp文件中的内容复制过来,注意,<form/>元素的action属性值要与处理器方法courseAddPojo的映射路径一致,给出对应代码。下一步,在“WEB-INF/pages/course”目录中,创建course_info_pojo.jsp文件,以EL方式显示请求域中的对象,给出对应代码。下一步,在index.jsp文件中,添加跳转至处理器方法toCourseAddPojo的链接,给出对应代码。 在com.javaee.ex12.po包中创建实体类Book,包含String类型的bname,pub分别对应教材名称,出版社。float类型的price对应价格。
时间: 2023-07-15 22:10:45 浏览: 131
对应代码如下:
add_course_basic.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>添加课程基本信息</title>
</head>
<body>
<h2>添加课程基本信息</h2>
<form action="${pageContext.request.contextPath}/course/addPojo" method="post">
课程编号:<input type="text" name="cid"><br>
课程名称:<input type="text" name="cname"><br>
学时数:<input type="text" name="period"><br>
教材名称:<input type="text" name="bname"><br>
出版社:<input type="text" name="pub"><br>
价格:<input type="text" name="price"><br>
<input type="submit" value="提交">
</form>
</body>
</html>
```
course_info_basic.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>课程信息</title>
</head>
<body>
<h2>课程信息</h2>
<p>课程编号:${course.cid}</p>
<p>课程名称:${course.cname}</p>
<p>学时数:${course.period}</p>
<p>教材名称:${course.bname}</p>
<p>出版社:${course.pub}</p>
<p>价格:${course.price}</p>
</body>
</html>
```
Course.java:
```
package com.javaee.ex12.po;
public class Course {
private String cid;
private String cname;
private String period;
private String bname;
private String pub;
private String price;
public String getCid() {
return cid;
}
public void setCid(String cid) {
this.cid = cid;
}
public String getCname() {
return cname;
}
public void setCname(String cname) {
this.cname = cname;
}
public String getPeriod() {
return period;
}
public void setPeriod(String period) {
this.period = period;
}
public String getBname() {
return bname;
}
public void setBname(String bname) {
this.bname = bname;
}
public String getPub() {
return pub;
}
public void setPub(String pub) {
this.pub = pub;
}
public String getPrice() {
return price;
}
public void setPrice(String price) {
this.price = price;
}
}
```
CourseController.java:
```
package com.javaee.ex12.controller;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.ModelAndView;
import com.javaee.ex12.po.Course;
@Controller
@RequestMapping("/course")
public class CourseController {
@RequestMapping("/toAddPojo")
public String toCourseAddPojo() {
return "course/add_course_pojo";
}
@RequestMapping("/addPojo")
public ModelAndView CourseAddPojo(HttpServletRequest request, HttpServletResponse response,
@ModelAttribute("course") Course course) {
ModelAndView mav = new ModelAndView();
mav.addObject("course", course);
mav.setViewName("course/course_info_pojo");
return mav;
}
}
```
add_course_pojo.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>添加课程基本信息</title>
</head>
<body>
<h2>添加课程基本信息</h2>
<form action="${pageContext.request.contextPath}/course/addPojo" method="post">
课程编号:<input type="text" name="cid"><br>
课程名称:<input type="text" name="cname"><br>
学时数:<input type="text" name="period"><br>
教材名称:<input type="text" name="bname"><br>
出版社:<input type="text" name="pub"><br>
价格:<input type="text" name="price"><br>
<input type="submit" value="提交">
</form>
</body>
</html>
```
course_info_pojo.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>课程信息</title>
</head>
<body>
<h2>课程信息</h2>
<p>课程编号:${course.cid}</p>
<p>课程名称:${course.cname}</p>
<p>学时数:${course.period}</p>
<p>教材名称:${course.bname}</p>
<p>出版社:${course.pub}</p>
<p>价格:${course.price}</p>
</body>
</html>
```
index.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
<h2>首页</h2>
<p><a href="${pageContext.request.contextPath}/course/toAddPojo">添加课程信息</a></p>
</body>
</html>
```
Book.java:
```
package com.javaee.ex12.po;
public class Book {
private String bname;
private String pub;
private float price;
public String getBname() {
return bname;
}
public void setBname(String bname) {
this.bname = bname;
}
public String getPub() {
return pub;
}
public void setPub(String pub) {
this.pub = pub;
}
public float getPrice() {
return price;
}
public void setPrice(float price) {
this.price = price;
}
}
```
相关推荐
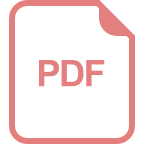
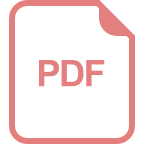















