Java 如何实现数据批量插入
时间: 2024-03-20 15:40:37 浏览: 38
Java 可以使用 JDBC(Java Database Connectivity)来实现数据批量插入。具体步骤如下:
1. 创建数据库连接并获取 Connection 对象。
2. 创建 PreparedStatement 对象,并使用 INSERT INTO 语句来定义要插入的数据表和字段。
3. 使用 PreparedStatement 的 addBatch() 方法将多个插入语句添加到批处理中。
4. 使用 executeBatch() 方法执行批处理,并将返回的结果存储在一个 int 数组中。
5. 释放资源,包括关闭 PreparedStatement 和 Connection 对象。
下面是一个示例代码:
```java
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection(url, username, password);
String sql = "INSERT INTO mytable (col1, col2, col3) VALUES (?, ?, ?)";
pstmt = conn.prepareStatement(sql);
for (int i = 0; i < dataList.size(); i++) {
pstmt.setString(1, dataList.get(i).getCol1());
pstmt.setString(2, dataList.get(i).getCol2());
pstmt.setString(3, dataList.get(i).getCol3());
pstmt.addBatch();
}
int[] result = pstmt.executeBatch();
} catch (SQLException e) {
e.printStackTrace();
} finally {
if (pstmt != null) {
try {
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们使用 PreparedStatement 的 addBatch() 方法来将多个插入语句添加到批处理中,然后使用 executeBatch() 方法执行批处理,并将返回的结果存储在一个 int 数组中。最后,我们释放资源,包括关闭 PreparedStatement 和 Connection 对象。
阅读全文
相关推荐
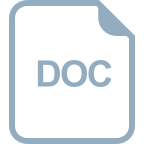
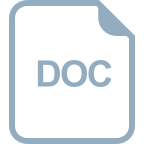
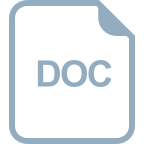
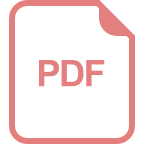
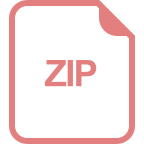
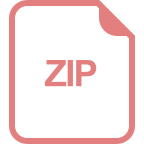
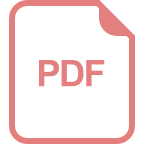










