#include <iostream>#include <vector>#include <stack>using namespace std;void dfs(int u, vector<vector<int>>& graph, vector<bool>& visited, vector<int>& farthest) { stack<int> s; s.push(u); visited[u] = true; while (!s.empty()) { int v = s.top(); s.pop(); for (int i = 0; i < graph[v].size(); i++) { int w = graph[v][i]; if (!visited[w]) { visited[w] = true; s.push(w); farthest[w] = farthest[v] + 1; } } }}int main() { int n, m; cin >> n >> m; vector<vector<int>> graph(n); for (int i = 0; i < m; i++) { int u, v; cin >> u >> v; graph[u].push_back(v); } vector<int> farthest(n, 0); for (int i = 0; i < n; i++) { vector<bool> visited(n, false); dfs(i, graph, visited, farthest); } for (int i = 0; i < n; i++) { cout << "Node " << i << " can reach farthest node " << farthest[i] << endl; } return 0;}不用STL实现这个代码
时间: 2024-01-13 17:05:22 浏览: 31
不使用STL实现该代码,可以手动实现栈和vector。
下面是C++实现:
```c++
#include <iostream>
#include <vector>
using namespace std;
const int MAXN = 10005;
int stack[MAXN], top = -1;
void push(int x) {
stack[++top] = x;
}
int pop() {
return stack[top--];
}
bool empty() {
return top == -1;
}
void dfs(int u, int graph[][MAXN], bool visited[], int farthest[]) {
push(u);
visited[u] = true;
while (!empty()) {
int v = pop();
for (int i = 0; i < MAXN; i++) {
if (graph[v][i] && !visited[i]) {
visited[i] = true;
push(i);
farthest[i] = farthest[v] + 1;
}
}
}
}
int main() {
int n, m;
cin >> n >> m;
int graph[MAXN][MAXN] = {0};
for (int i = 0; i < m; i++) {
int u, v;
cin >> u >> v;
graph[u][v] = 1;
}
int farthest[MAXN] = {0};
for (int i = 0; i < n; i++) {
bool visited[MAXN] = {false};
dfs(i, graph, visited, farthest);
}
for (int i = 0; i < n; i++) {
cout << "Node " << i << " can reach farthest node " << farthest[i] << endl;
}
return 0;
}
```
在这个实现中,我们手动实现了栈,并使用数组来代替vector。同时,我们使用二维数组来表示邻接矩阵,而不是vector<vector<int>>来表示邻接表。
其他部分的实现与使用STL的版本相同。
相关推荐
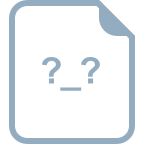














