c语言实现将文本通过uart传输
时间: 2024-11-12 14:34:21 浏览: 15
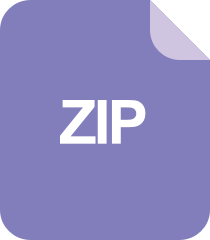
通过UART串口实现的helloworld程序.zip
在C语言中,要通过UART(通用异步接收发送)接口将文本数据传输,通常需要以下几个步骤:
1. **包含头文件**:首先,你需要包含相关的硬件接口头文件,如`stdio.h`(用于处理字符串)和`serial.h`或`sys/ioctl.h`(取决于你的平台和库支持)。
```c
#include <stdio.h>
#include <unistd.h> // Linux下的IO操作
#include <fcntl.h>
```
2. **打开设备**:使用`open()`函数打开UART设备文件,例如 `/dev/ttyUSB0`(假设是USB串口),并获取描述符。
```c
int uart_fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
if (uart_fd < 0) {
perror("Error opening UART");
return -1;
}
```
3. **设置波特率**:如果需要,可以使用`tcgetattr()`、`cfsetispeed()` 和 `cfsetospeed()` 来设置波特率和其他通信参数。
4. **发送数据**:将文本转换为字符数组,然后使用`write()`函数将数据写入设备。这里是一个简单的例子:
```c
char text[] = "Hello, UART!";
size_t bytes_sent = write(uart_fd, text, strlen(text) + 1); // 加一表示包括结束符'\0'
if (bytes_sent <= 0) {
perror("Error writing to UART");
close(uart_fd);
return -1;
}
```
5. **关闭设备**:当完成传输后,记得关闭打开的设备文件。
```c
close(uart_fd);
```
阅读全文
相关推荐
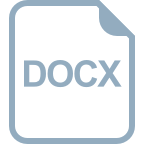
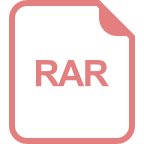

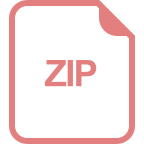
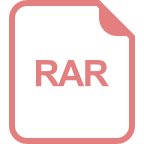
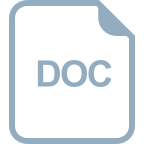
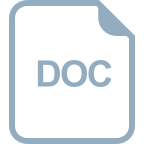
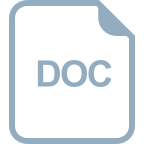
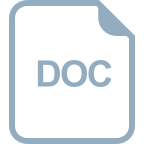
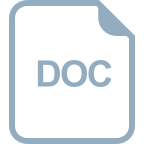
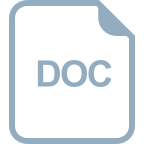
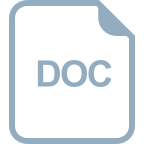
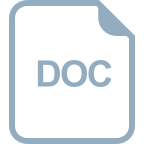
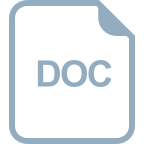
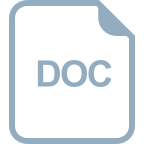
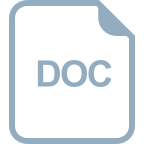
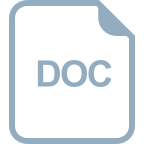