struct chexinxi *addchexinxi() { char str[]="0"; struct chexinxi *p,*pr; struct chexinxi *head; pr=p=(struct chexinxi *)malloc(sizeof(struct chexinxi)); printf("\t\t\t\t请输入以下内容(车牌号或车型号为0时退出):\n"); printf("\t\t\t\t车型号:"); scanf("%s",p->chexinghao); printf("\t\t\t\t车牌号:"); scanf("%s",p->chepaihao); printf("\n"); head=NULL; while(1) { if(strcmp(p->chexinghao,str)!=0&&strcmp(p->chepaihao,str)!=0) { if(head==NULL) head=p; else { pr->next=p; pr=p; } p=(struct chexinxi *)malloc(sizeof(struct chexinxi)); printf("\t\t\t\t车型号:"); scanf("%s",p->chexinghao); printf("\t\t\t\t车牌号:"); scanf("%s",p->chepaihao); printf("\n"); } else break; } pr->next=NULL; return head; }优化代码写入文件
时间: 2023-11-02 10:04:56 浏览: 38
首先,需要定义一个文件指针,打开一个文件以便将数据写入文件中。然后,在代码中加入文件写入操作即可。
以下是优化后的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct chexinxi {
char chexinghao[20];
char chepaihao[20];
struct chexinxi *next;
};
struct chexinxi *addchexinxi() {
char str[]="0";
struct chexinxi *p,*pr;
struct chexinxi *head;
pr=p=(struct chexinxi *)malloc(sizeof(struct chexinxi));
printf("\t\t\t\t请输入以下内容(车牌号或车型号为0时退出):\n");
printf("\t\t\t\t车型号:");
scanf("%s",p->chexinghao);
printf("\t\t\t\t车牌号:");
scanf("%s",p->chepaihao);
printf("\n");
// 打开文件
FILE *fp = fopen("data.txt", "w");
if (fp == NULL) {
printf("Failed to open file!\n");
exit(1);
}
head=NULL;
while(1) {
if(strcmp(p->chexinghao,str)!=0&&strcmp(p->chepaihao,str)!=0) {
if(head==NULL)
head=p;
else {
pr->next=p;
pr=p;
}
p=(struct chexinxi *)malloc(sizeof(struct chexinxi));
// 将数据写入文件中
fprintf(fp, "%s %s\n", pr->chexinghao, pr->chepaihao);
printf("\t\t\t\t车型号:");
scanf("%s",p->chexinghao);
printf("\t\t\t\t车牌号:");
scanf("%s",p->chepaihao);
printf("\n");
}
else
break;
}
pr->next=NULL;
// 关闭文件
fclose(fp);
return head;
}
int main() {
addchexinxi();
return 0;
}
```
在上述代码中,我们使用 `fopen()` 函数打开了一个名为 `data.txt` 的文件,并使用 `fprintf()` 函数将每次输入的车型号和车牌号写入文件中。最后使用 `fclose()` 函数关闭文件。
注意,在打开文件之前,需要进行错误检查,以确保文件是否成功打开。如果文件打开失败,则应该退出程序。
相关推荐
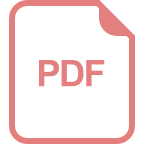
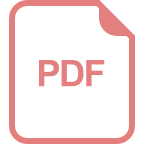
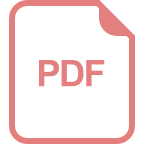















