Python双人五子棋代码
时间: 2023-12-25 22:29:33 浏览: 35
以下是一个使用Python编写的双人五子棋游戏的示例代码:
```python
import tkinter as tk
# 创建棋盘
def create_board():
board = []
for _ in range(15):
row = []
for _ in range(15):
row.append(0)
board.append(row)
return board
# 判断是否获胜
def check_win(board, row, col, player):
# 水平方向
count = 0
for i in range(col-4, col+5):
if i < 0 or i >= 15:
continue
if board[row][i] == player:
count += 1
if count == 5:
return True
else:
count = 0
# 垂直方向
count = 0
for i in range(row-4, row+5):
if i < 0 or i >= 15:
continue
if board[i][col] == player:
count += 1
if count == 5:
return True
else:
count = 0
# 左上到右下方向
count = 0
for i in range(-4, 5):
r = row + i
c = col + i
if r < 0 or r >= 15 or c < 0 or c >= 15:
continue
if board[r][c] == player:
count += 1
if count == 5:
return True
else:
count = 0
# 右上到左下方向
count = 0
for i in range(-4, 5):
r = row + i
c = col - i
if r < 0 or r >= 15 or c < 0 or c >= 15:
continue
if board[r][c] == player:
count += 1
if count == 5:
return True
else:
count = 0
return False
# 下棋
def play(row, col):
global current_player, board
if board[row][col] == 0:
board[row][col] = current_player
if check_win(board, row, col, current_player):
print("玩家", current_player, "获胜!")
restart_game()
else:
current_player = 3 - current_player
# 重新开始游戏
def restart_game():
global current_player, board
board = create_board()
current_player = 1
# 创建GUI界面
def create_gui():
window = tk.Tk()
window.title("双人五子棋")
window.geometry("450x450")
canvas = tk.Canvas(window, width=450, height=450)
canvas.pack()
for i in range(15):
canvas.create_line(30, 30+i*30, 420, 30+i*30)
canvas.create_line(30+i*30, 30, 30+i*30, 420)
def click(event):
col = (event.x - 15) // 30
row = (event.y - 15) // 30
play(row, col)
canvas.create_oval(col*30+5, row*30+5, col*30+25, row*30+25, fill="black" if current_player == 1 else "white")
canvas.bind("<Button-1>", click)
restart_button = tk.Button(window, text="重新开始", command=restart_game)
restart_button.pack()
window.mainloop()
board = create_board()
current_player = 1
create_gui()
```
相关推荐
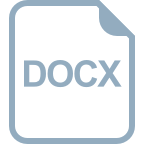
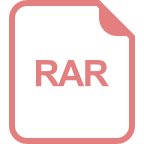














