pygame双人五子棋代码
时间: 2023-07-03 09:28:22 浏览: 62
以下是一个基于Pygame实现的双人五子棋游戏代码:
```python
import pygame
import numpy as np
# 初始化Pygame
pygame.init()
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
BLUE = (0, 0, 255)
GREEN = (0, 255, 0)
# 定义棋盘大小和每个格子的大小
BOARD_SIZE = (600, 600)
GRID_SIZE = 40
# 创建窗口
screen = pygame.display.set_mode(BOARD_SIZE)
pygame.display.set_caption("双人五子棋")
# 创建一个15x15的棋盘
board = np.zeros((15, 15), dtype=int)
# 定义棋子落下的函数
def put_stone(x, y, stone):
board[x][y] = stone
# 定义判断胜负的函数
def check_win(x, y, stone):
# 判断横向是否有五子相连
count = 0
for i in range(15):
if board[x][i] == stone:
count += 1
if count == 5:
return True
else:
count = 0
# 判断纵向是否有五子相连
count = 0
for i in range(15):
if board[i][y] == stone:
count += 1
if count == 5:
return True
else:
count = 0
# 判断左上到右下是否有五子相连
count = 0
for i in range(-4, 5):
if 0 <= x+i < 15 and 0 <= y+i < 15 and board[x+i][y+i] == stone:
count += 1
if count == 5:
return True
else:
count = 0
# 判断左下到右上是否有五子相连
count = 0
for i in range(-4, 5):
if 0 <= x+i < 15 and 0 <= y-i < 15 and board[x+i][y-i] == stone:
count += 1
if count == 5:
return True
else:
count = 0
return False
# 绘制棋盘
def draw_board():
# 绘制棋盘背景
screen.fill(WHITE)
# 绘制棋盘网格
for i in range(15):
pygame.draw.line(screen, BLACK, (GRID_SIZE, i*GRID_SIZE+GRID_SIZE), (GRID_SIZE*14, i*GRID_SIZE+GRID_SIZE), 2)
pygame.draw.line(screen, BLACK, (i*GRID_SIZE+GRID_SIZE, GRID_SIZE), (i*GRID_SIZE+GRID_SIZE, GRID_SIZE*14), 2)
# 绘制棋盘上已有的棋子
for i in range(15):
for j in range(15):
if board[i][j] == 1:
pygame.draw.circle(screen, BLACK, (i*GRID_SIZE+GRID_SIZE, j*GRID_SIZE+GRID_SIZE), 18, 0)
elif board[i][j] == 2:
pygame.draw.circle(screen, WHITE, (i*GRID_SIZE+GRID_SIZE, j*GRID_SIZE+GRID_SIZE), 18, 0)
# 定义主程序
def main():
# 定义当前下棋的人
current_player = 1
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
if event.type == pygame.MOUSEBUTTONDOWN:
# 获取鼠标点击的位置
x, y = pygame.mouse.get_pos()
# 将鼠标点击的位置转换为棋子位置
x = int((x - GRID_SIZE/2) / GRID_SIZE)
y = int((y - GRID_SIZE/2) / GRID_SIZE)
# 判断该位置是否已经有棋子
if board[x][y] != 0:
continue
# 落子
put_stone(x, y, current_player)
# 判断是否胜利
if check_win(x, y, current_player):
print("玩家{}获胜!".format(current_player))
pygame.quit()
quit()
# 切换下棋的人
current_player = 2 if current_player == 1 else 1
# 绘制棋盘和棋子
draw_board()
pygame.display.update()
if __name__ == '__main__':
main()
```
这个代码基于Pygame实现了一个简单的双人五子棋游戏,界面比较简洁,但是可以实现基本的落子和判断胜负功能。玩家可以通过鼠标点击来落子,当有一方获胜时游戏结束。
相关推荐
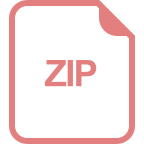
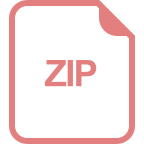










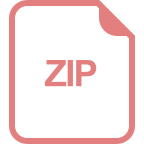
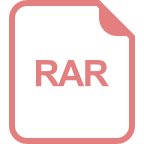