unity 单例模式的实现
时间: 2024-05-12 10:11:14 浏览: 12
在Unity中,单例模式是一种常用的设计模式,用于确保一个类只有一个实例,并提供全局访问点。以下是Unity中实现单例模式的一种常见方式:
```csharp
public class SingletonExample : MonoBehaviour
{
private static SingletonExample instance;
// 私有构造函数,防止外部实例化
private SingletonExample() { }
// 全局访问点,返回单例实例
public static SingletonExample Instance
{
get
{
if (instance == null)
{
instance = FindObjectOfType<SingletonExample>();
if (instance == null)
{
GameObject singletonObject = new GameObject();
instance = singletonObject.AddComponent<SingletonExample>();
singletonObject.name = "SingletonExample";
DontDestroyOnLoad(singletonObject);
}
}
return instance;
}
}
// 其他方法和属性...
}
```
上述代码中,SingletonExample类的构造函数被声明为私有,这样外部无法直接实例化该类。通过静态属性Instance来获取单例实例,如果实例不存在,则会在场景中查找该类的实例,如果仍不存在,则会创建一个新的GameObject并添加该类的实例组件,并将其设置为不销毁的对象。
相关推荐
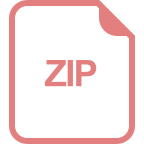
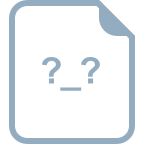














