编写一个图书类(Book)和一个图书测试类(TestBook)
时间: 2024-10-15 07:07:43 浏览: 34
好的,我们可以创建一个简单的图书类(Book)作为基础实体,并编写一个测试类(TestBook)来进行相关的功能测试。
**图书类(Book.java):**
```java
public class Book {
private String title;
private String author;
private int publicationYear;
public Book(String title, String author, int publicationYear) {
this.title = title;
this.author = author;
this.publicationYear = publicationYear;
}
// getter and setter methods
public String getTitle() { return title; }
public void setTitle(String title) { this.title = title; }
public String getAuthor() { return author; }
public void setAuthor(String author) { this.author = author; }
public int getPublicationYear() { return publicationYear; }
public void setPublicationYear(int publicationYear) { this.publicationYear = publicationYear; }
@Override
public String toString() {
return "Title: " + title + ", Author: " + author + ", Year: " + publicationYear;
}
}
```
**图书测试类(TestBook.java):**
```java
import org.junit.Test;
public class TestBook {
@Test
public void testBookProperties() {
Book book1 = new Book("The Catcher in the Rye", "J.D. Salinger", 1951);
System.out.println(book1); // 测试标题、作者和年份是否正确显示
// 检查默认构造函数
Book book2 = new Book();
assert book2.getTitle().equals("");
// 测试setter和getter方法
book2.setTitle("To Kill a Mockingbird");
book2.setAuthor("Harper Lee");
book2.setPublicationYear(1960);
System.out.println(book2);
}
}
```
在这个例子中,`Book` 类代表基本的图书信息,而 `TestBook` 类则是用来测试 `Book` 类的各种方法是否正常工作的单元测试类。`TestBook` 中的 `testBookProperties` 方法用于验证书名、作者和出版年份属性的设置和获取功能。
阅读全文
相关推荐
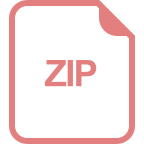
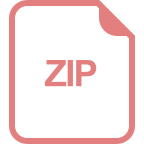
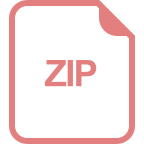




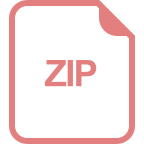
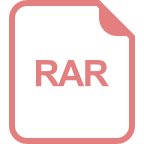
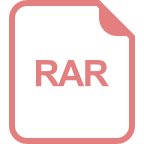
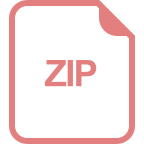





