用c++编写实现顺序表的初始化、插入、删除、输出、判空、判满、求值及查找等操作
时间: 2024-09-29 16:04:32 浏览: 34
在C++中,我们可以使用数组结构来创建一个简单的顺序表(也称为线性表),通常用于教学目的。以下是基本的操作实现:
```cpp
// 定义顺序表节点
struct Node {
int data;
Node* next;
};
// 初始化顺序表(长度为0)
class SequenceList {
private:
Node* head;
int size;
public:
SequenceList() : head(nullptr), size(0) {}
// 判断表是否为空
bool isEmpty() const { return head == nullptr; }
// 插入元素到列表末尾
void insert(int value) {
if (size == MAX_SIZE) { // 如果已满
throw std::runtime_error("Sequence list is full");
}
Node* newNode = new Node{value, nullptr};
if (head == nullptr) { // 空表插入
head = newNode;
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
size++;
}
// 删除指定位置的元素(假设索引从0开始)
void remove(int index) {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
if (index == 0) { // 删除头节点
Node* temp = head;
head = head->next;
delete temp;
} else {
Node* prev = head;
for (int i = 1; i < index; ++i) {
prev = prev->next;
}
Node* curr = prev->next;
prev->next = curr->next;
delete curr;
}
size--;
}
// 输出整个列表
void print() const {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << "\n";
}
// 查找元素并返回其位置(找不到返回-1)
int find(int value) const {
Node* current = head;
for (int i = 0; i < size && current != nullptr; ++i, current = current->next) {
if (current->data == value) {
return i;
}
}
return -1;
}
};
// 注意:这里MAX_SIZE是一个常量,可以根据实际需要调整顺序表的最大容量
const int MAX_SIZE = 100;
```
阅读全文
相关推荐
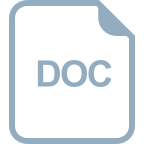
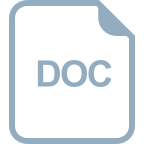
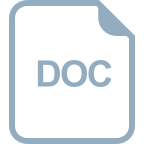



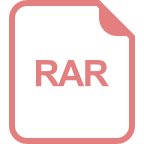











