用c语言代码写出输入字符串str和字符ch,输出ch在str中最后一次出现的位置;若没出现,则输出0。字符串长度不超过50。
时间: 2024-05-15 13:19:05 浏览: 9
好的,我明白了。下面是C语言代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[51], ch;
int len, i, pos = 0;
printf("请输入字符串:");
scanf("%s", str);
printf("请输入字符:");
scanf(" %c", &ch);
len = strlen(str);
for (i = 0; i < len; i++) {
if (str[i] == ch) {
pos = i + 1;
}
}
if (pos == 0) {
printf("该字符在字符串中未出现!\n");
} else {
printf("该字符最后一次出现的位置是:%d\n", pos);
}
return 0;
}
```
希望能解答你的问题!
相关问题
C语言代码:输入字符串str和字符ch,输出ch在str中最后一次出现的位置;若没出现,则输出0。字符串长度不超过50。 输入格式: 输入包括两行。 第一行输入字符串str 第二行输入字符ch 输出格式: 输出ch在str中出现的最后一次,若没有则输出0.
好的,我明白你的要求了。以下是解答:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char str[51], ch;
int len, i, last = 0;
// 输入字符串和字符
scanf("%s", str);
scanf(" %c", &ch);
// 获取字符串长度
len = strlen(str);
// 查找字符
for (i = 0; i < len; i++) {
if (str[i] == ch) {
last = i + 1; // 记录位置
}
}
// 输出最后一次出现的位置或0
printf("%d\n", last);
return 0;
}
```
以上就是 C 语言代码,输入一个字符串和一个字符,输出字符在字符串中最后一次出现的位置。如果该字符没有出现过,则输出0。
希望我的解答能够帮到你。如果你还有其他问题,请随时问我。
在c语言中,编写一个程序,输入一个字符串str和一个字符ch,通过指针查找,统计字符串str中ch出现的位置
下面是一个实现该功能的C语言程序:
```c
#include <stdio.h>
#include <string.h>
void find_char(const char *str, char ch, int *pos, int *count) {
int len = strlen(str);
*count = 0;
for (int i = 0; i < len; i++) {
if (str[i] == ch) {
pos[*count] = i;
(*count)++;
}
}
}
int main() {
char str[100];
char ch;
int pos[100];
int count = 0;
printf("请输入一个字符串:");
scanf("%s", str);
printf("请输入要查找的字符:");
scanf(" %c", &ch);
find_char(str, ch, pos, &count);
if (count == 0) {
printf("字符串中未找到该字符。\n");
} else {
printf("该字符在字符串中出现了%d次,位置分别为:\n", count);
for (int i = 0; i < count; i++) {
printf("%d ", pos[i]);
}
printf("\n");
}
return 0;
}
```
在程序中,我们定义了一个`find_char`函数,它接收一个字符串、一个字符、一个位置数组和一个计数器指针作为输入,通过指针查找字符串中指定字符的位置,并将位置存储在位置数组中,最后更新计数器指针的值为该字符在字符串中出现的次数。
在`main`函数中,我们先读入一个字符串和一个字符,然后调用`find_char`函数查找该字符在字符串中的位置,最后输出结果。
需要注意的是,在调用`scanf`函数读入字符时,我们需要在前面加上一个空格,以消耗掉之前输入时留下的换行符或空格。
相关推荐
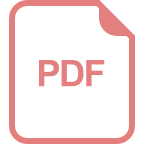
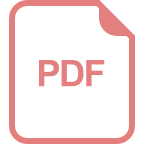
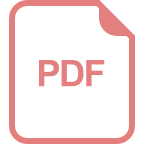












