#include <bits/stdc++.h> using namespace std; typedef string ElemType; typedef struct BitNode { ElemType data; struct BitNode *lchild,*rchild; } BitNode,*BiTree; //char sc[10]= {"+-*/"}; void CreateBiTree(BiTree &t) { string ch; cin >> ch; if(ch[0] == '#') t = NULL; else { t = new BitNode; t->data = ch; CreateBiTree(t->lchild); CreateBiTree(t->rchild); } } void InOrderTraverse(BiTree t) { if(t) { string op = t->data; if(op[0]<'0' || op[0]>'9') cout << '('; InOrderTraverse(t->lchild); cout << t->data ; InOrderTraverse(t->rchild); if(op[0]<'0' || op[0]>'9') cout << ')'; } /***********************************/ } int main() { BiTree tree = new BitNode; string ch; while(cin >> ch) { tree->data = ch; CreateBiTree(tree->lchild); CreateBiTree(tree->rchild); InOrderTraverse(tree); printf("\n"); } return 0; }换为c语言
时间: 2024-03-17 19:44:29 浏览: 27
把代码中的头文件改为标准C语言的头文件,去掉using namespace std,把string改为char数组,去掉注释,把printf改为printf_s(或者使用头文件stdio.h中的printf函数)等等,修改后的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef char ElemType;
typedef struct BitNode {
ElemType data;
struct BitNode *lchild,*rchild;
} BitNode,*BiTree;
void CreateBiTree(BiTree *t) {
char ch;
scanf("%c", &ch);
if(ch == '#')
*t = NULL;
else
{
*t = (BitNode*)malloc(sizeof(BitNode));
(*t)->data = ch;
CreateBiTree(&((*t)->lchild));
CreateBiTree(&((*t)->rchild));
}
}
void InOrderTraverse(BiTree t) {
if(t) {
char op = t->data;
if(op < '0' || op > '9') {
printf("(");
}
InOrderTraverse(t->lchild);
printf("%c", t->data);
InOrderTraverse(t->rchild);
if(op < '0' || op > '9') {
printf(")");
}
}
}
int main() {
BiTree tree = NULL;
char ch;
while(scanf("%c", &ch) != EOF) {
tree = (BitNode*)malloc(sizeof(BitNode));
tree->data = ch;
CreateBiTree(&(tree->lchild));
CreateBiTree(&(tree->rchild));
InOrderTraverse(tree);
printf_s("\n");
}
return 0;
}
```
相关推荐
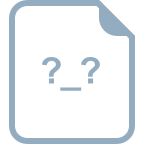
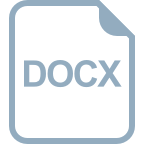
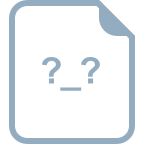

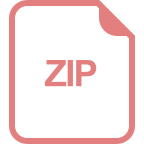
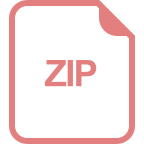
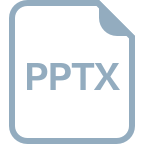
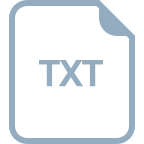
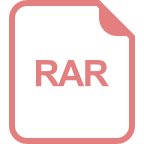