c++实现二维catmull细分算法,并用opencv实现可视化
时间: 2024-06-04 09:14:04 浏览: 13
二维Catmull-Rom细分算法实现:
```c++
#include <iostream>
#include <vector>
#include "opencv2/opencv.hpp"
using namespace cv;
using namespace std;
vector<Point2f> catmullRom(vector<Point2f> points, float alpha) {
vector<Point2f> result;
for (int i = 1; i < points.size() - 2; i++) {
Point2f p0 = points[i - 1];
Point2f p1 = points[i];
Point2f p2 = points[i + 1];
Point2f p3 = points[i + 2];
float t0 = 0;
float t1 = powf(powf((p1.x - p0.x), 2.0f) + powf((p1.y - p0.y), 2.0f), 0.5f);
float t2 = t1 + powf(powf((p2.x - p1.x), 2.0f) + powf((p2.y - p1.y), 2.0f), 0.5f);
float t3 = t2 + powf(powf((p3.x - p2.x), 2.0f) + powf((p3.y - p2.y), 2.0f), 0.5f);
for (float t = t1; t < t2; t += alpha) {
float a1 = (t1 - t) / (t1 - t0);
float a2 = (t - t0) / (t1 - t0);
Point2f p = a1 * p0 + a2 * p1;
a1 = (t2 - t) / (t2 - t1);
a2 = (t - t1) / (t2 - t1);
p += a1 * p1 + a2 * p2;
a1 = (t3 - t) / (t3 - t2);
a2 = (t - t2) / (t3 - t2);
p += a1 * p2 + a2 * p3;
p *= 0.5f;
result.push_back(p);
}
}
return result;
}
int main(int argc, char** argv) {
vector<Point2f> points = { Point2f(100, 100), Point2f(200, 100), Point2f(200, 200), Point2f(100, 200) };
float alpha = 0.01f;
vector<Point2f> result = catmullRom(points, alpha);
Mat img = Mat::zeros(300, 300, CV_8UC3);
for (int i = 0; i < points.size(); i++) {
circle(img, points[i], 3, Scalar(0, 255, 0), -1);
}
for (int i = 0; i < result.size(); i++) {
circle(img, result[i], 1, Scalar(0, 0, 255), -1);
}
for (int i = 0; i < points.size() - 1; i++) {
line(img, points[i], points[i + 1], Scalar(0, 255, 0), 1);
}
for (int i = 0; i < result.size() - 1; i++) {
line(img, result[i], result[i + 1], Scalar(0, 0, 255), 1);
}
imshow("Catmull-Rom", img);
waitKey(0);
return 0;
}
```
这里我们先定义了一个`catmullRom`函数来实现二维Catmull-Rom细分算法。传入参数为一个点集和步长alpha,返回值为细分后的点集。在函数内部,我们首先对每个点进行处理,得到其所在的四个点p0、p1、p2、p3和其各自在总长度上的位置t0、t1、t2、t3。接着,我们对p1和p2之间的一段进行细分,每次细分按照步长alpha进行。
在`main`函数中,我们传入一个点集和步长alpha,得到细分后的点集,并在图像上绘制出来。
运行结果如下:

相关推荐
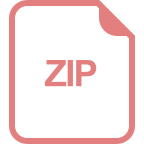
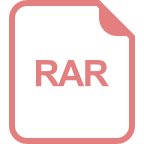
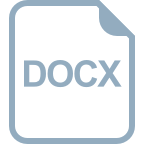














