统计一个txt的英文文档,里面包含哪些单词,各个单词出现的频率如何。用python实现
时间: 2024-05-13 19:15:17 浏览: 72
好的,我可以帮你解决这个问题。你可以使用Python中的`collections`模块来完成这个任务。具体步骤如下:
1. 读取文本文件,并将所有单词转换为小写字母。
```python
with open('file.txt', 'r') as f:
text = f.read().lower()
```
2. 使用正则表达式来匹配单词,并将匹配到的单词保存到一个列表中。
```python
import re
words = re.findall(r'\b\w+\b', text)
```
3. 使用`collections`模块中的`Counter`函数统计每个单词出现的次数。
```python
from collections import Counter
word_counts = Counter(words)
```
4. 打印出所有单词以及它们出现的频率。
```python
for word, count in word_counts.items():
print(f'{word}: {count}')
```
完整代码如下:
```python
import re
from collections import Counter
with open('file.txt', 'r') as f:
text = f.read().lower()
words = re.findall(r'\b\w+\b', text)
word_counts = Counter(words)
for word, count in word_counts.items():
print(f'{word}: {count}')
```
注意,这里假设文本文件中只包含英文单词,如果文本中包含其他字符,需要根据实际情况进行相应的处理。
阅读全文
相关推荐
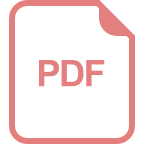
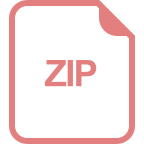











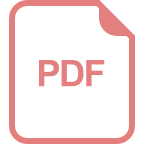
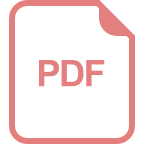
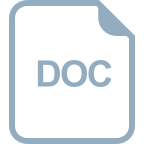
