python统计一个txt的英文文档,里面包含哪些单词,各个单词出现的频率如何
时间: 2024-05-15 12:17:37 浏览: 122
您可以使用Python中的nltk库进行文本处理。首先,您需要读取该文件并将其转换为小写。然后您可以使用nltk中的word_tokenize函数将文本分成单词,并使用nltk.FreqDist函数计算每个单词出现的频率。以下是实现此任务的示例代码:
```python
import nltk
from nltk.tokenize import word_tokenize
from nltk.probability import FreqDist
# 读取文本文件
with open("your_file.txt", "r") as f:
text = f.read()
# 把文本转换成小写
text = text.lower()
# 分词词
words = word_tokenize(text)
# 计算每个单词出现的频率
freq_dist = FreqDist(words)
# 输出每个单词和它们的频率
for word, frequency in freq_dist.items():
print(f"{word}: {frequency}")
```
运行此代码后,您将获得一个单词列表和每个单词的频率。
相关问题
用python统计一个txt的英文文档,里面包含哪些单词,各个单词出现的频率如何
可以用Python的内置模块collections中的Counter函数来实现。
首先需要读取文本文件,可以使用Python的内置模块open来打开文件并读取内容。
```python
with open('example.txt', 'r') as f:
text = f.read()
```
接下来需要将文本中的单词进行分割并统计出现的频率,可以使用Python的split函数和collections模块中的Counter函数来实现。
```python
import collections
words = text.split()
word_count = collections.Counter(words)
```
最后可以输出每个单词以及它出现的频率。
```python
for word, count in word_count.most_common():
print(word, count)
```
完整代码如下:
```python
import collections
with open('example.txt', 'r') as f:
text = f.read()
words = text.split()
word_count = collections.Counter(words)
for word, count in word_count.most_common():
print(word, count)
```
Python统计一个txt的英文文档,里面包含哪些单词,各个单词出现的频率如何。
可以用Python中的文件读取和字符串处理模块来实现文本的读取和单词的统计。
以下是一个简单的程序示例:
```python
import string
# 打开文件并读取内容
with open('example.txt', 'r') as f:
content = f.read()
# 去除标点符号并转换为小写
content = content.translate(str.maketrans('', '', string.punctuation)).lower()
# 将文本分割为单词列表
words = content.split()
# 统计单词出现的频率
word_freq = {}
for word in words:
if word in word_freq:
word_freq[word] += 1
else:
word_freq[word] = 1
# 输出结果
for word, freq in word_freq.items():
print(word, freq)
```
解释一下上面的代码:
1. 使用 `with open()` 语句打开文件并读取文件内容到变量 `content` 中。
2. 使用 `str.translate()` 方法去除标点符号,并使用 `str.lower()` 方法转换为小写字母。
3. 使用 `str.split()` 方法将文本分割为单词列表。
4. 遍历单词列表,统计每个单词出现的频率,保存到字典 `word_freq` 中。
5. 遍历 `word_freq` 字典,输出每个单词和对应的频率。
注意,上述程序只是一个简单的示例,对于一些特殊情况(如单词中包含连字符、缩写词等),还需要进行特殊处理。
阅读全文
相关推荐
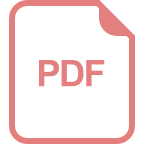
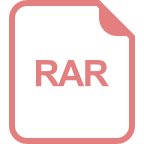





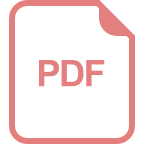





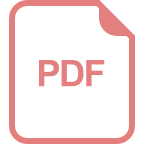
