C++,设计学生信息的类,包含数据成员学号、姓名和成绩(语文、数学和英语)和总成绩,其中总成绩是各成绩之和。写出完整的类定义,并通过主函数中定义不同对象并实现该对象的信息输入、计算与输出。
时间: 2024-11-03 16:21:15 浏览: 49
在C++中,你可以创建一个名为`Student`的类来表示学生的相关信息。这个类将包含私有数据成员如学号、姓名、单科成绩以及一个计算总成绩的方法。以下是完整的类定义:
```cpp
#include <iostream>
using namespace std;
class Student {
private:
int id; // 学号
string name; // 姓名
int chineseScore; // 语文成绩
int mathScore; // 数学成绩
int englishScore; // 英语成绩
public:
// 构造函数
Student(int id, string name, int cs, int ms, int es) : id(id), name(name), chineseScore(cs), mathScore(ms), englishScore(es) {}
// 计算总成绩的方法
int calculateTotalScore() {
return chineseScore + mathScore + englishScore;
}
// 获取和设置方法
void setInput(int id, string name, int cs, int ms, int es) {
this->id = id;
this->name = name;
this->chineseScore = cs;
this->mathScore = ms;
this->englishScore = es;
}
// 输出信息的方法
void displayInfo() {
cout << "学号: " << id << endl;
cout << "姓名: " << name << endl;
cout << "语文成绩: " << chineseScore << endl;
cout << "数学成绩: " << mathScore << endl;
cout << "英语成绩: " << englishScore << endl;
cout << "总成绩: " << calculateTotalScore() << endl;
}
};
int main() {
// 创建对象并输入信息
Student stu1(1001, "张三", 85, 90, 87);
stu1.setInput(1002, "李四", 92, 88, 93);
// 显示学生信息
stu1.displayInfo();
// 主函数结束
return 0;
}
```
在这个例子中,`main`函数中定义了两个`Student`对象`stu1`和`stu2`,分别设置了他们的基本信息,然后通过`displayInfo()`方法输出了各自的信息。每个学生对象有自己的总成绩计算方式,体现了多态性的一个简单应用。
阅读全文
相关推荐
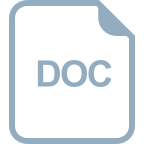
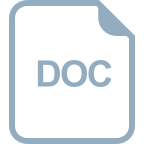
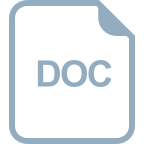















