商品库存管理系统c++
时间: 2023-09-28 18:06:51 浏览: 107
商品库存管理系统是一个常见的商业应用程序,它可以帮助商家追踪他们的库存情况、销售情况和库存成本等。下面是一个简单的C++程序示例,可以实现基本的商品库存管理功能。
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 商品类
class Product {
public:
Product(string name, int quantity, double price) {
this->name = name;
this->quantity = quantity;
this->price = price;
}
string getName() {
return name;
}
int getQuantity() {
return quantity;
}
double getPrice() {
return price;
}
void setQuantity(int quantity) {
this->quantity = quantity;
}
private:
string name; // 商品名称
int quantity; // 商品数量
double price; // 商品价格
};
// 商品库存管理类
class Inventory {
public:
// 添加商品
void addProduct(Product product) {
products.push_back(product);
}
// 删除商品
void removeProduct(int index) {
if (index >= 0 && index < products.size()) {
products.erase(products.begin() + index);
}
}
// 获取商品数量
int getProductCount() {
return products.size();
}
// 获取商品
Product getProduct(int index) {
if (index >= 0 && index < products.size()) {
return products[index];
}
}
// 更新商品数量
void updateProductQuantity(int index, int quantity) {
if (index >= 0 && index < products.size()) {
products[index].setQuantity(quantity);
}
}
private:
vector<Product> products; // 商品列表
};
int main() {
// 创建商品库存管理对象
Inventory inventory;
// 添加商品
inventory.addProduct(Product("商品1", 10, 10.0));
inventory.addProduct(Product("商品2", 20, 20.0));
// 获取商品数量
cout << "商品数量: " << inventory.getProductCount() << endl;
// 获取商品
Product product = inventory.getProduct(0);
cout << "商品名称: " << product.getName() << endl;
cout << "商品价格: " << product.getPrice() << endl;
// 更新商品数量
inventory.updateProductQuantity(0, 5);
product = inventory.getProduct(0);
cout << "更新后商品数量: " << product.getQuantity() << endl;
// 删除商品
inventory.removeProduct(0);
cout << "删除后商品数量: " << inventory.getProductCount() << endl;
return 0;
}
```
这个程序演示了如何创建一个简单的商品库存管理系统,通过Product和Inventory类来处理商品信息和库存信息。你可以根据自己的需求来扩展这个程序,比如添加更多的商品属性、实现更复杂的库存管理功能等。
阅读全文
相关推荐

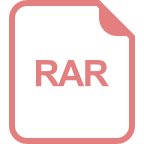
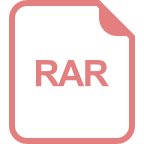
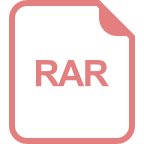


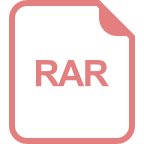





