this.canvas = new fabric.Canvas 禁止鼠标移动到canvas的范围外
时间: 2023-08-12 10:42:44 浏览: 47
要禁止鼠标移动到canvas的范围外,您可以添加`mousemove`事件监听器,检查鼠标位置是否超出canvas的范围,并在超出范围时阻止默认的鼠标移动行为。以下是一个示例代码:
```
this.canvas.on('mousemove', function(e) {
var canvas = e.target;
var boundingRect = canvas.getBoundingClientRect();
// 检查鼠标位置是否超出canvas范围
if (e.clientX < boundingRect.left || e.clientX > boundingRect.right || e.clientY < boundingRect.top || e.clientY > boundingRect.bottom) {
e.preventDefault();
}
});
```
在这个示例代码中,我们获取canvas的边界矩形,并检查鼠标位置是否超出了这个矩形范围。如果超出范围,我们就阻止默认的鼠标移动行为。这样就可以禁止鼠标移动到canvas的范围外了。
相关问题
如何实时的对this.rectangle = new fabric.Rect监控,禁止rect的坐标,超出canvas的大小范围
您可以使用fabric.js提供的`object:moving`事件来监控矩形对象的移动,并在移动时检查其位置是否超出了canvas的边界。可以在`object:moving`事件处理程序中添加以下代码:
```
this.rectangle.on('object:moving', function(e) {
var obj = e.target;
var canvas = obj.canvas;
var boundingRect = obj.getBoundingRect();
// 检查是否超出左上角边界
if (boundingRect.top < 0 || boundingRect.left < 0) {
obj.top = Math.max(obj.top, 0);
obj.left = Math.max(obj.left, 0);
obj.setCoords();
}
// 检查是否超出右下角边界
if (boundingRect.top + boundingRect.height > canvas.height || boundingRect.left + boundingRect.width > canvas.width) {
obj.top = Math.min(obj.top, canvas.height - boundingRect.height);
obj.left = Math.min(obj.left, canvas.width - boundingRect.width);
obj.setCoords();
}
});
```
这段代码会在矩形对象移动时监控其位置,并在其位置超出canvas的边界时将其强制限制在canvas范围内。
拉扯的过程当中,不允许this.rectangle = new fabric.Rect的坐标 不允许 超过canvas的高度和宽度
要确保当对象被拉伸时不会超出画布的边界,您可以在 `object:scaling` 事件中检查对象的位置和大小,然后根据需要调整其坐标。
以下是一个示例代码,它将在对象被拉伸时防止其超出画布的大小:
```javascript
this.canvas.on('object:scaling', function(e) {
var obj = e.target;
var canvasWidth = this.width;
var canvasHeight = this.height;
var objWidth = obj.getScaledWidth() * obj.scaleX;
var objHeight = obj.getScaledHeight() * obj.scaleY;
// 确定对象在X轴和Y轴上的最小和最大位置
var objLeft = obj.left - objWidth / 2;
var objRight = objLeft + objWidth;
var objTop = obj.top - objHeight / 2;
var objBottom = objTop + objHeight;
// 如果对象超出了画布的大小,则阻止缩放
if (objLeft < 0 || objTop < 0 || objRight > canvasWidth || objBottom > canvasHeight) {
// 计算对象的新坐标
var newLeft = Math.min(Math.max(obj.left, objWidth / 2), canvasWidth - objWidth / 2);
var newTop = Math.min(Math.max(obj.top, objHeight / 2), canvasHeight - objHeight / 2);
// 重置对象的位置
obj.set({
left: newLeft,
top: newTop,
scaleX: obj.scaleX,
scaleY: obj.scaleY
});
obj.setCoords();
}
});
```
这段代码将在对象被缩放时触发,并检查对象是否超出了画布的大小。如果是,则计算对象的新坐标,并将其设置为新的位置。请注意,这里使用了Math.min和Math.max函数来确保对象不会超出画布的边界。
相关推荐
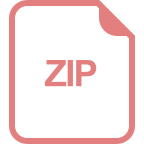
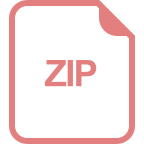











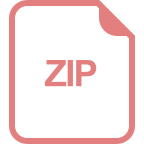
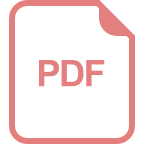