c++如何返回字符串数组
时间: 2024-02-01 11:15:02 浏览: 228
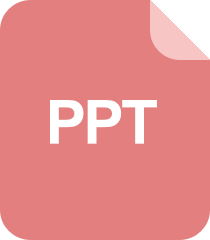
字符串查找(t-初级字符串
在 C++ 中,可以使用以下两种方式返回字符串数组:
1. 通过指针返回字符串数组
```c++
#include <iostream>
#include <string>
using namespace std;
// 返回字符串数组的函数
string* getStrings() {
static string strs[] = {"hello", "world", "how", "are", "you"};
return strs;
}
int main() {
// 调用函数获取字符串数组
string* strs = getStrings();
// 输出字符串数组
for (int i = 0; i < 5; i++) {
cout << strs[i] << endl;
}
return 0;
}
```
2. 通过引用参数返回字符串数组
```c++
#include <iostream>
#include <string>
using namespace std;
// 返回字符串数组的函数
void getStrings(string (&strs)[5]) {
strs[0] = "hello";
strs[1] = "world";
strs[2] = "how";
strs[3] = "are";
strs[4] = "you";
}
int main() {
// 定义字符串数组
string strs[5];
// 调用函数获取字符串数组
getStrings(strs);
// 输出字符串数组
for (int i = 0; i < 5; i++) {
cout << strs[i] << endl;
}
return 0;
}
```
注意:在第一种方式中,需要使用静态变量来存储字符串数组,否则在返回后该数组会被销毁。在第二种方式中,需要使用引用参数来传递字符串数组,否则仍然会复制整个数组,而不是返回指向数组的指针。
阅读全文
相关推荐
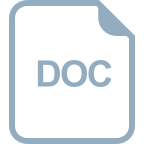
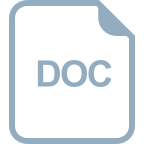



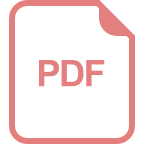
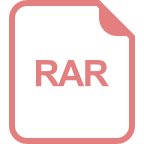
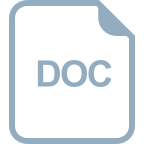
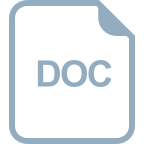
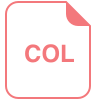
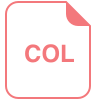





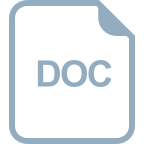
