Please write a genetic algorithm in Python to calculate the maximum value of function f (x)=0.4+sinc (4x)+1.1sinc (4x+2)+0.8sinc (X-2)+0.7sinc (6x-4) between -2 and 2. The function solution is represented by a 16 bit binary number. The population size is 30, the crossover probability is 0.3, the mutation probability is 0.01, and the number of iterations is 400
时间: 2024-03-27 07:41:20 浏览: 89
Sure, here's an implementation of the genetic algorithm you described:
```python
import random
import math
def fitness_function(x):
return 0.4 + math.sin(4 * x) / (4 * x) + 1.1 * math.sin(4 * x + 2) / (4 * x + 2) + \
0.8 * math.sin(x - 2) / (x - 2) + 0.7 * math.sin(6 * x - 4) / (6 * x - 4)
def binary_to_float(binary_num):
return -2 + (4 / (2 ** 16 - 1)) * int(binary_num, 2)
def generate_population(population_size):
population = []
for i in range(population_size):
individual = ''.join(random.choice(['0', '1']) for _ in range(16))
population.append(individual)
return population
def selection(population):
fitness_scores = [fitness_function(binary_to_float(individual)) for individual in population]
total_fitness = sum(fitness_scores)
probabilities = [fitness / total_fitness for fitness in fitness_scores]
selected = random.choices(population, probabilities, k=2)
return selected[0], selected[1]
def crossover(parent1, parent2, crossover_prob):
if random.random() < crossover_prob:
crossover_point = random.randint(1, 14)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
else:
return parent1, parent2
def mutation(individual, mutation_prob):
mutated = ''
for bit in individual:
if random.random() < mutation_prob:
mutated += '0' if bit == '1' else '1'
else:
mutated += bit
return mutated
def genetic_algorithm(population_size, crossover_prob, mutation_prob, num_iterations):
population = generate_population(population_size)
for i in range(num_iterations):
new_population = []
for j in range(population_size // 2):
parent1, parent2 = selection(population)
child1, child2 = crossover(parent1, parent2, crossover_prob)
child1 = mutation(child1, mutation_prob)
child2 = mutation(child2, mutation_prob)
new_population.extend([child1, child2])
population = new_population
best_individual = max(population, key=lambda x: fitness_function(binary_to_float(x)))
return binary_to_float(best_individual)
```
You can call this function with the parameters you specified to get the maximum value of the given function:
```python
result = genetic_algorithm(population_size=30, crossover_prob=0.3, mutation_prob=0.01, num_iterations=400)
print(result)
```
Note that the solution may not always be the same due to the random nature of the genetic algorithm.
阅读全文
相关推荐
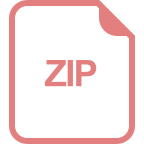
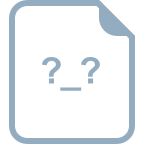
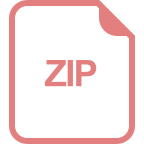


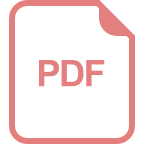
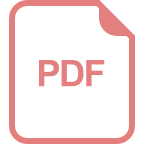
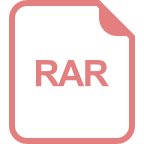
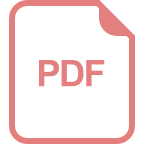
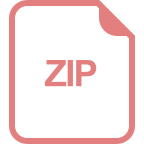
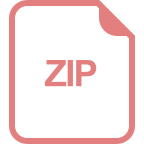
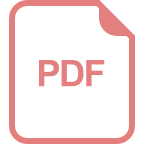
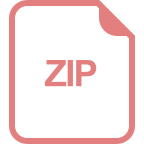
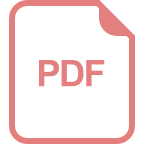
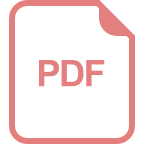
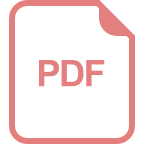
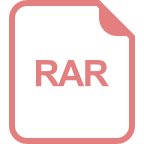