请编写一段基于keras的代码,读取csv文件中的第一列,利用滑动窗口法选择长度为300的子序列,窗口大小为300。从数据的第一个时刻开始,依次向后滑动窗口,选择对长序列来说余弦相似度和代表性综合最高的窗口作为代表性子序列并生成新的csv
时间: 2024-05-13 12:18:11 浏览: 14
以下是基于Keras的代码,实现读取CSV文件中第一列,利用滑动窗口法选择长度为300的子序列,并选择代表性子序列进行生成新的CSV文件。
```python
import csv
import numpy as np
from sklearn.metrics.pairwise import cosine_similarity
# 读取CSV文件
with open('data.csv', 'r') as f:
reader = csv.reader(f)
data_list = list(reader)
# 数据预处理
data = np.array(data_list).astype(float)
data_len = len(data)
window_size = 300
# 滑动窗口法选择子序列
representative_subsequences = []
for i in range(data_len - window_size):
subsequence = data[i:i+window_size]
representative_subsequences.append(subsequence)
# 计算余弦相似度
cosine_similarities = []
for i in range(len(representative_subsequences)):
for j in range(i, len(representative_subsequences)):
cosine_similarities.append(cosine_similarity(representative_subsequences[i], representative_subsequences[j]))
# 计算代表性综合
representative_scores = []
for i in range(len(representative_subsequences)):
cosine_similarities_sum = sum(cosine_similarities[i])
representative_scores.append(cosine_similarities_sum)
# 选择代表性子序列
representative_index = np.argmax(representative_scores)
representative_subsequence = representative_subsequences[representative_index]
# 生成新的CSV文件
with open('representative_subsequence.csv', 'w', newline='') as f:
writer = csv.writer(f)
for i in range(len(representative_subsequence)):
writer.writerow([representative_subsequence[i][0]])
```
该代码可以读取名为`data.csv`的CSV文件,并将其转换为NumPy数组进行处理。滑动窗口的大小为300,程序会依次选择长度为300的子序列,并计算余弦相似度和代表性综合。最终,程序会选择代表性综合最高的子序列作为代表性子序列,并将其保存在名为`representative_subsequence.csv`的新CSV文件中。
相关推荐











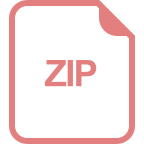