边缘跟踪c++代码(不利于OpenCV与MATLAB)
时间: 2023-12-08 08:05:48 浏览: 61
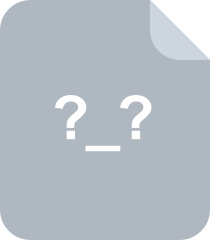
边缘检测c++代码

以下是使用C++实现的边缘跟踪算法代码示例:
```c++
#include <iostream>
#include <cmath>
using namespace std;
// 获取像素值
int get_pixel_value(int x, int y, int width, unsigned char *input) {
if (x < 0 || y < 0 || x >= width || y >= width) {
return 0;
}
return input[y * width + x];
}
// 边缘跟踪
void edge_tracing(int height, int width, unsigned char *input, unsigned char *output) {
// 阈值
int threshold = 50;
// 方向模板
int dir_x[8] = {-1, 0, 1, 1, 1, 0, -1, -1};
int dir_y[8] = {1, 1, 1, 0, -1, -1, -1, 0};
// 遍历像素
for (int y = 0; y < height; ++y) {
for (int x = 0; x < width; ++x) {
// 获取像素值
int pixel = input[y * width + x];
// 判断是否为边缘点
if (pixel >= threshold) {
// 判断周围8个像素是否为边缘点
bool is_edge = false;
for (int i = 0; i < 8; ++i) {
int nx = x + dir_x[i];
int ny = y + dir_y[i];
int npixel = get_pixel_value(nx, ny, width, input);
if (npixel >= threshold) {
is_edge = true;
break;
}
}
// 如果周围8个像素中有边缘点,则该点为边缘点
if (is_edge) {
output[y * width + x] = 255;
}
}
}
}
}
int main() {
// 读入图像
int width = 512, height = 512;
unsigned char *input = new unsigned char[width * height];
unsigned char *output = new unsigned char[width * height];
FILE *fp = fopen("lena.raw", "rb");
fread(input, sizeof(unsigned char), width * height, fp);
fclose(fp);
// 边缘跟踪
edge_tracing(height, width, input, output);
// 输出图像
FILE *fout = fopen("lena_edge_tracing.raw", "wb");
fwrite(output, sizeof(unsigned char), width * height, fout);
fclose(fout);
delete[] input;
delete[] output;
return 0;
}
```
该代码使用边缘跟踪算法对图像进行边缘提取,并将结果输出到文件中。其中,`edge_tracing`函数对每个像素进行判断是否为边缘点,`get_pixel_value`函数获取指定位置像素的值。
阅读全文
相关推荐
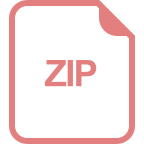
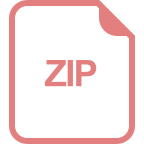
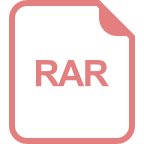
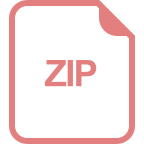
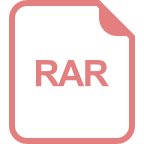
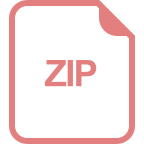
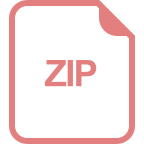
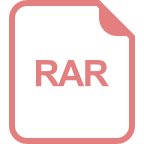
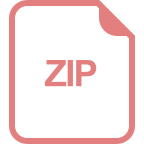
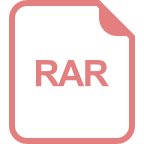
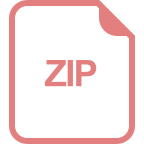
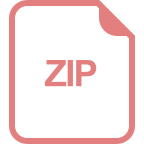
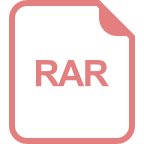
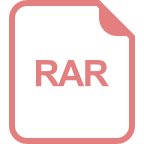
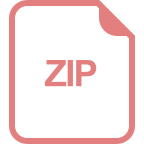
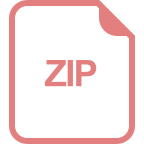