单词词频python
时间: 2023-11-08 18:05:58 浏览: 71
可以使用Python中的collections模块中的Counter类来统计单词词频。具体实现步骤如下:
1. 读取文本文件内容
2. 将文本内容转换为小写字母
3. 使用正则表达式去除标点符号和数字
4. 将文本内容按照空格分割成单词列表
5. 使用Counter类统计单词出现次数
以下是示例代码:
```python
import re
from collections import Counter
with open('text.txt', 'r') as f:
text = f.read()
text = text.lower()
text = re.sub(r'[^\w\s]', '', text)
text = re.sub(r'\d+', '', text)
words = text.split()
word_counts = Counter(words)
print(word_counts.most_common(10))
```
以上代码将输出出现频率最高的前10个单词及其出现次数。
相关问题
统计词频python
在Python中,统计词频可以使用内置的数据结构如字典(dict),结合循环和条件判断,也可以使用专门用于文本处理的库如NLTK (Natural Language Toolkit) 或者 `collections` 中的 `Counter` 类。这里是一个简单的例子,使用Python和`collections.Counter` 来统计一个字符串或文件中单词的频率:
```python
from collections import Counter
def count_words(text):
# 删除标点符号并将所有字母转为小写
text = ''.join(e for e in text if e.isalnum() or e.isspace()).lower()
# 分割文本成单词
words = text.split()
# 使用Counter计算词频
word_counts = Counter(words)
return word_counts
# 示例,统计字符串中的词频
text = "这是一段测试文本,它包含了一些重复的词语。"
word_freqs = count_words(text)
print(word_freqs)
# 或者统计文件中的词频
with open('yourfile.txt', 'r', encoding='utf-8') as file:
text = file.read()
word_freqs = count_words(text)
print(word_freqs)
```
运行这段代码后,你会得到一个字典,其中键是单词,值是该单词在文本中出现的次数。
mapreduce词频python
在Python中使用MapReduce模式计算词频可以很方便地实现。下面是一个简单的示例代码:
```python
from collections import Counter
from multiprocessing import Pool
def mapper(text):
words = text.split()
return Counter(words)
def reducer(counters):
return sum(counters, Counter())
def map_reduce(data, num_processes):
pool = Pool(num_processes)
mapped_data = pool.map(mapper, data)
reduced_data = reducer(mapped_data)
pool.close()
pool.join()
return reduced_data
if __name__ == "__main__":
# 假设有一些文本数据
data = [
"Hello world",
"Hello CSDN",
"CSDN is great",
"Hello python"
]
# 使用MapReduce计算词频
word_count = map_reduce(data, 2)
# 输出结果
for word, count in word_count.items():
print(f"{word}: {count}")
```
运行以上代码,你将会得到每个单词出现的次数。这是一个简单的MapReduce实现,你可以根据你的需求进行修改和扩展。
阅读全文
相关推荐
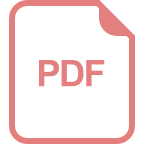
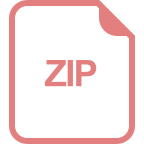
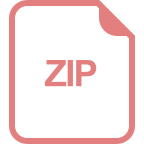
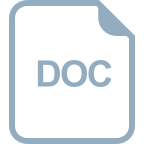
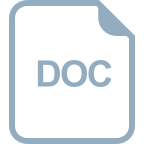








