c++ std::map insert用法
时间: 2024-10-20 13:13:05 浏览: 14
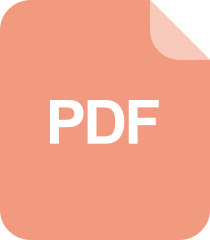
基于C++ map中key使用指针问题的详解
在C++中,`std::map` 是 C++ Standard Library 提供的一个关联容器,它存储键值对,并自动排序键(默认升序)。如果你想插入一个新的元素到 `std::map` 中,可以使用 `insert` 函数。基本语法如下:
```cpp
#include <map>
using namespace std;
// 假设 map 存储整数和字符串,key_type是int,value_type是string
map<int, string> myMap;
// 插入新元素,如果key已经存在则不会添加新的条目
myMap.insert({ key, value });
// 或者使用更具体的版本,返回一个迭代器指向新插入的位置
pair<iterator, bool> result = myMap.insert(make_pair(key, value));
if (result.second) {
// 插入成功,value_type的第一个元素(在这里是string)会被赋给iterator的引用
// 迭代器指向新插入的元素
}
```
如果你尝试插入的键已经存在,`insert` 函数会直接返回现有键对应的迭代器,`bool` 成员表示插入是否成功(true表示新插入,false表示已存在)。
阅读全文
相关推荐
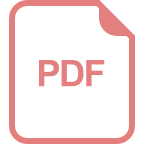
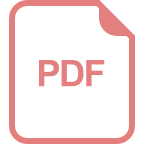















